Understanding PCB Programming
Printed Circuit Board (PCB) programming is the process of writing software code and configuring hardware components to enable a PCB to perform specific functions. PCBs are essential components in various electronic devices, from smartphones and computers to industrial machinery and medical equipment. In this article, we will explore the fundamentals of PCB programming, the tools and techniques used, and the steps involved in the process.
What is a PCB?
A PCB is a flat board made of insulating material, such as fiberglass or plastic, with conductive tracks, pads, and other features etched onto its surface. These conductive elements are used to electrically connect and mechanically support various electronic components, such as resistors, capacitors, and integrated circuits (ICs). PCBs are designed using specialized software called Electronic Design Automation (EDA) tools, which help engineers create schematics, layout designs, and generate manufacturing files.
Why is PCB Programming Important?
PCB programming is crucial because it enables the PCB to perform its intended functions. Without proper programming, a PCB is just a collection of components without any purpose. Programming allows engineers to define the behavior of the PCB, control its inputs and outputs, and implement complex algorithms and protocols. It also enables the PCB to communicate with other devices, process data, and make decisions based on predefined conditions.
PCB Programming Tools and Techniques
Hardware Description Languages (HDLs)
Hardware Description Languages (HDLs) are specialized programming languages used to describe the behavior and structure of electronic circuits. The two most common HDLs are Verilog and VHDL (Very High-Speed Integrated Circuit Hardware Description Language). These languages allow engineers to write code that defines the functionality of the PCB at a high level of abstraction, which can then be synthesized into a lower-level representation for implementation on the actual hardware.
Verilog
Verilog is a popular HDL used in the design and verification of digital circuits. It was developed by Gateway Design Automation in 1985 and has since become an IEEE standard. Verilog uses a C-like syntax and supports both behavioral and structural descriptions of circuits. It allows engineers to model complex systems using modules, which can be connected together to form larger designs.
VHDL
VHDL is another widely used HDL, developed by the U.S. Department of Defense in the early 1980s. Like Verilog, VHDL is an IEEE standard and supports both behavioral and structural descriptions of circuits. However, VHDL has a more verbose syntax compared to Verilog and is often preferred for its strong typing and extensive libraries.
Integrated Development Environments (IDEs)
Integrated Development Environments (IDEs) are software applications that provide a comprehensive set of tools for writing, debugging, and simulating HDL code. IDEs offer features such as syntax highlighting, code completion, and integrated debugging, which greatly simplify the PCB programming process. Some popular IDEs for PCB programming include:
Xilinx Vivado
Xilinx Vivado is a powerful IDE for designing and programming PCBs based on Xilinx FPGAs (Field Programmable Gate Arrays) and SoCs (System-on-Chips). It supports both Verilog and VHDL and offers a wide range of tools for design entry, synthesis, simulation, and implementation.
Intel Quartus Prime
Intel Quartus Prime is an IDE for designing and programming PCBs based on Intel FPGAs and SoCs. It supports both Verilog and VHDL and provides a comprehensive set of tools for design entry, synthesis, simulation, and optimization.
Mentor Graphics HDL Designer
Mentor Graphics HDL Designer is an IDE for creating, simulating, and verifying digital designs using Verilog, VHDL, and SystemVerilog. It offers a user-friendly interface, powerful debugging capabilities, and integration with other Mentor Graphics tools for PCB design and analysis.
Simulation and Verification
Before a PCB can be programmed and manufactured, it is essential to simulate and verify its functionality to ensure that it meets the design requirements and specifications. Simulation allows engineers to test the PCB’s behavior under various conditions and identify potential issues early in the design process. Verification involves comparing the simulated results with the expected outcomes to ensure that the PCB functions correctly.
Several tools and techniques are used for PCB simulation and verification, including:
ModelSim
ModelSim is a popular HDL simulator that supports Verilog, VHDL, and SystemVerilog. It allows engineers to simulate and debug their designs at various levels of abstraction, from individual modules to entire systems. ModelSim offers features such as waveform viewing, code coverage analysis, and assertion-based verification.
Synopsys VCS
Synopsys VCS is a high-performance HDL simulator that supports Verilog, VHDL, and SystemVerilog. It offers advanced verification capabilities, such as constrained random testing, functional coverage analysis, and low-power simulation. VCS is widely used in the semiconductor industry for the verification of complex digital designs.
Cadence Incisive
Cadence Incisive is a comprehensive suite of tools for the functional verification of digital designs. It includes an HDL simulator, formal verification tools, and a unified debug environment. Incisive supports Verilog, VHDL, and SystemVerilog and offers features such as multi-language simulation, mixed-signal simulation, and hardware-software co-verification.
PCB Programming Process
The PCB programming process involves several steps, from design entry to implementation and testing. The following is a typical workflow for PCB programming:
-
Design Entry: The first step in PCB programming is to create a schematic diagram of the circuit using an EDA tool. The schematic captures the logical connections between the components and defines the overall functionality of the PCB.
-
HDL Coding: Once the schematic is complete, the next step is to write HDL code that describes the behavior of the circuit. This involves defining the inputs, outputs, and internal signals of the PCB, as well as the logic that governs its operation. The HDL code is typically written in Verilog or VHDL using an IDE.
-
Simulation: After the HDL code is written, it is simulated using an HDL simulator to verify its functionality. The simulator applies test stimuli to the inputs of the PCB and observes the outputs to ensure that they match the expected results. Any issues or bugs identified during simulation are fixed before proceeding to the next step.
-
Synthesis: Once the HDL code is verified through simulation, it is synthesized into a lower-level representation, such as a netlist or a bitstream. Synthesis involves mapping the high-level HDL code to the physical resources of the target device, such as the logic gates, flip-flops, and memory elements of an FPGA.
-
Place and Route: After synthesis, the next step is to place and route the design onto the physical device. This involves assigning the synthesized logic to specific locations on the device and connecting them using the available routing resources. The place and route process is typically automated using specialized algorithms and tools.
-
Bitstream Generation: Once the design is placed and routed, a bitstream is generated that contains the configuration data for the target device. The bitstream is essentially a binary file that tells the device how to configure its hardware resources to implement the desired functionality.
-
Programming: The final step is to program the target device with the generated bitstream. This is typically done using a programming cable or a JTAG (Joint Test Action Group) interface, which allows the bitstream to be loaded into the device’s configuration memory. Once programmed, the PCB is ready to be tested and deployed in the final application.
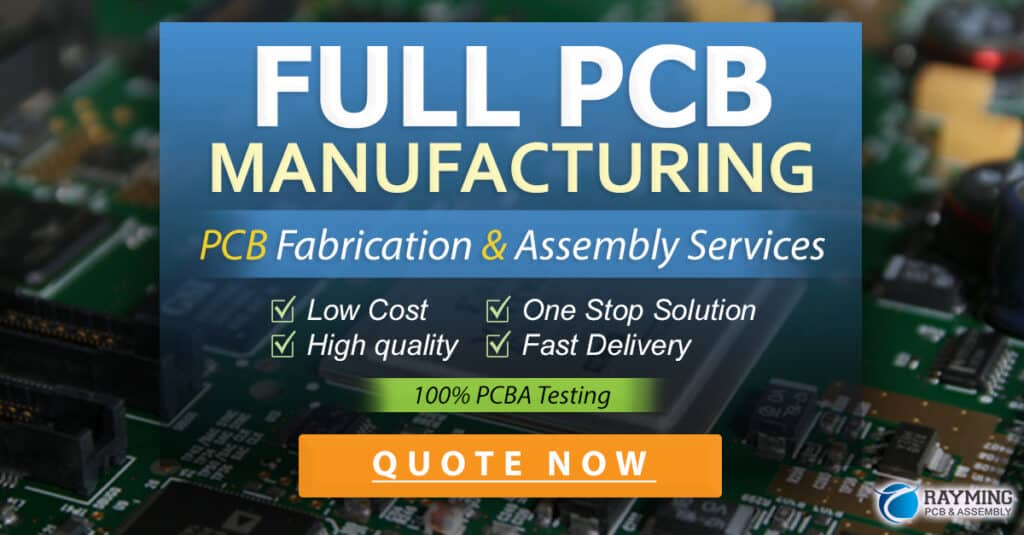
PCB Programming Example
To illustrate the PCB programming process, let’s consider a simple example of a 4-bit binary counter implemented on an FPGA. The counter will increment its value by one on each rising edge of a clock signal and display the current count on four LED outputs.
Step 1: Design Entry
The first step is to create a schematic diagram of the counter using an EDA tool. The schematic will include the following components:
- A clock input
- A reset input
- Four LED outputs
- A 4-bit register to store the current count
- An incrementer to add one to the current count on each clock edge
Step 2: HDL Coding
Next, we write the HDL code that describes the behavior of the counter. The following is an example Verilog code for the 4-bit binary counter:
module binary_counter(
input clk,
input reset,
output [3:0] count
);
reg [3:0] count_reg;
always @(posedge clk or posedge reset) begin
if (reset) begin
count_reg <= 4'b0000;
end else begin
count_reg <= count_reg + 1;
end
end
assign count = count_reg;
endmodule
In this code, we define a module named binary_counter
with a clock input (clk
), a reset input (reset
), and a 4-bit output (count
). We declare a 4-bit register (count_reg
) to store the current count value. The always
block is triggered on the rising edge of the clock or the reset signal. If the reset signal is asserted, the count register is cleared to zero. Otherwise, the count register is incremented by one on each clock edge. Finally, the value of the count register is assigned to the output port.
Step 3: Simulation
After writing the HDL code, we simulate it using an HDL simulator to verify its functionality. We create a testbench that generates a clock signal and a reset signal, and we observe the count output to ensure that it increments correctly on each clock edge and resets to zero when the reset signal is asserted.
Step 4: Synthesis
Once the HDL code is verified through simulation, we synthesize it into a netlist using a synthesis tool. The synthesis tool maps the Verilog code to the specific hardware resources available on the target FPGA, such as lookup tables (LUTs), flip-flops, and routing resources.
Step 5: Place and Route
After synthesis, we use a place and route tool to assign the synthesized logic to specific locations on the FPGA and connect them using the available routing resources. This process is automated and takes into account various constraints, such as timing, power, and area.
Step 6: Bitstream Generation
Once the design is placed and routed, we generate a bitstream that contains the configuration data for the FPGA. The bitstream is a binary file that tells the FPGA how to configure its hardware resources to implement the 4-bit binary counter.
Step 7: Programming
Finally, we program the FPGA with the generated bitstream using a programming cable or a JTAG interface. Once programmed, the FPGA will function as a 4-bit binary counter, incrementing the count value on each clock edge and displaying it on the four LED outputs.
Frequently Asked Questions (FAQ)
-
What is the difference between Verilog and VHDL?
Verilog and VHDL are both hardware description languages used for designing and programming PCBs. Verilog has a C-like syntax and is more concise, while VHDL has a more verbose syntax and is strongly typed. Verilog is often preferred for its simplicity and ease of use, while VHDL is favored for its extensive libraries and strong typing. -
What is an FPGA, and how is it different from a microcontroller?
An FPGA (Field Programmable Gate Array) is a type of programmable logic device that can be configured to implement custom hardware designs. Unlike a microcontroller, which has a fixed architecture and runs software, an FPGA can be programmed to create custom hardware circuits. FPGAs offer higher performance and flexibility compared to microcontrollers but are also more complex and expensive. -
What is the purpose of simulation in PCB programming?
Simulation is an essential step in PCB programming that allows engineers to verify the functionality of their designs before implementing them on actual hardware. By simulating the HDL code using test stimuli, engineers can identify and fix bugs, optimize performance, and ensure that the PCB meets the design requirements. Simulation helps to reduce the risk of errors and saves time and cost in the long run. -
What is the role of synthesis in PCB programming?
Synthesis is the process of converting the high-level HDL code into a lower-level representation that can be mapped onto the physical resources of the target device, such as an FPGA. During synthesis, the HDL code is optimized and translated into a netlist, which specifies the connections between the various logic elements of the device. Synthesis is a critical step in PCB programming that bridges the gap between the abstract design and the actual hardware implementation. -
What are some common challenges in PCB programming?
Some common challenges in PCB programming include: - Ensuring proper synchronization and timing of signals
- Managing power consumption and heat dissipation
- Dealing with signal integrity issues, such as crosstalk and noise
- Verifying the functionality of complex designs with multiple interacting components
- Debugging and troubleshooting hardware issues during testing and deployment
To overcome these challenges, engineers must follow best practices in PCB design, use appropriate tools and techniques for simulation and verification, and collaborate closely with other team members, such as hardware designers and test engineers.
Conclusion
PCB programming is a complex and multifaceted process that requires a deep understanding of electronic circuits, hardware description languages, and programming tools. By following a systematic approach that includes design entry, HDL coding, simulation, synthesis, place and route, bitstream generation, and programming, engineers can create highly functional and reliable PCBs for a wide range of applications.
As PCB technology continues to evolve, with higher speeds, lower power consumption, and greater integration, the challenges and opportunities in PCB programming will only grow. By staying up-to-date with the latest tools, techniques, and best practices, and by collaborating with other experts in the field, pcb programmers can continue to push the boundaries of what is possible and create innovative solutions that shape the future of electronics.
Step | Description | Tools |
---|---|---|
Design Entry | Create a schematic diagram of the circuit | EDA tools (e.g., Altium Designer, OrCAD, Eagle) |
HDL Coding | Write HDL code that describes the behavior of the circuit | IDEs (e.g., Xilinx Vivado, Intel Quartus Prime, Mentor Graphics HDL Designer) |
Simulation | Verify the functionality of the HDL code using test stimuli | HDL simulators (e.g., ModelSim, Synopsys VCS, Cadence Incisive) |
Synthesis | Convert the HDL code into a lower-level representation | Synthesis tools (e.g., Xilinx Vivado, Intel Quartus Prime) |
Place and Route | Assign the synthesized logic to specific locations on the device and connect them using routing resources | Place and route tools (e.g., Xilinx Vivado, Intel Quartus Prime) |
Bitstream Generation | Generate a binary file that contains the configuration data for the target device | Bitstream generation tools (e.g., Xilinx Vivado, Intel Quartus Prime) |
Programming | Load the bitstream into the target device’s configuration memory using a programming cable or JTAG interface | Programming tools (e.g., Xilinx Impact, Intel Quartus Prime Programmer) |
Leave a Reply