Introduction to Intelligent Power Switch
An Intelligent Power Switch is a device that can control the power supply to electronic devices based on predefined conditions or remote commands. It is particularly useful for managing the power consumption of Raspberry Pi, a popular single-board computer used in various applications such as home automation, robotics, and IoT projects. The Intelligent Power Switch can help optimize energy usage, protect the Raspberry Pi from power-related issues, and enable remote control of the device.
Benefits of using an Intelligent Power Switch with Raspberry Pi
-
Power optimization: The Intelligent Power Switch can monitor the power consumption of the Raspberry Pi and connected devices, allowing users to optimize energy usage and reduce electricity costs.
-
Protection against power issues: By controlling the power supply, the Intelligent Power Switch can protect the Raspberry Pi from power surges, undervoltage, or overvoltage conditions, preventing damage to the device.
-
Remote control: With an Intelligent Power Switch, users can remotely turn on or off the Raspberry Pi and connected devices, making it convenient for managing the device when physical access is not possible.
-
Automated power management: The Intelligent Power Switch can be programmed to automatically turn on or off the Raspberry Pi based on predefined conditions, such as time schedules or sensor data, enabling automated power management.
How Intelligent Power Switches work
An Intelligent Power Switch typically consists of the following components:
-
Power input: This is where the main power supply is connected to the Intelligent Power Switch.
-
Power output: The power output connects to the Raspberry Pi and other devices that need to be controlled.
-
Microcontroller: A microcontroller, such as an Arduino or ESP32, is used to control the power switching and monitor the power consumption.
-
Relay or MOSFET: A relay or MOSFET is used to physically switch the power on or off based on the microcontroller’s commands.
-
Sensors (optional): Various sensors, such as current sensors or temperature sensors, can be connected to the microcontroller to monitor the power consumption or environmental conditions.
The microcontroller in the Intelligent Power Switch runs a program that monitors the power consumption and other sensor data, and controls the relay or MOSFET to switch the power on or off based on predefined conditions or remote commands received through a communication interface (e.g., Wi-Fi, Bluetooth, or serial communication).
Example of an Intelligent Power Switch circuit
Component | Quantity | Description |
---|---|---|
Arduino Nano | 1 | Microcontroller to control the power switching |
Relay module | 1 | 5V relay module to switch the power on/off |
Current sensor | 1 | ACS712 current sensor to monitor power consumption |
Voltage regulator | 1 | LM7805 voltage regulator to provide stable 5V power |
Resistors, capacitors, and diodes | As needed | For proper circuit operation and protection |
The Arduino Nano reads the current sensor data and controls the relay module based on the predefined conditions or remote commands. The voltage regulator ensures a stable 5V power supply for the Arduino and relay module.
Configuring and using an Intelligent Power Switch
Setting up the hardware
- Connect the power input of the Intelligent Power Switch to a suitable power supply.
- Connect the power output of the Intelligent Power Switch to the Raspberry Pi and other devices that need to be controlled.
- If using sensors, connect them to the appropriate pins on the microcontroller according to the manufacturer’s instructions.
Programming the microcontroller
- Install the necessary development environment for the chosen microcontroller (e.g., Arduino IDE for Arduino boards).
- Write a program that reads sensor data, monitors power consumption, and controls the relay or MOSFET based on predefined conditions or remote commands.
- Upload the program to the microcontroller.
Example Arduino code for an Intelligent Power Switch:
#include <ACS712.h>
const int relayPin = 2;
const int currentSensorPin = A0;
ACS712 currentSensor(ACS712_05B, currentSensorPin);
void setup() {
pinMode(relayPin, OUTPUT);
Serial.begin(9600);
currentSensor.calibrate();
}
void loop() {
float current = currentSensor.getCurrentDC();
Serial.print("Current: ");
Serial.print(current);
Serial.println(" A");
if (current > 1.0) {
digitalWrite(relayPin, HIGH);
delay(5000);
digitalWrite(relayPin, LOW);
}
delay(1000);
}
This example code uses the ACS712 library to read current data from the current sensor. If the current exceeds 1A, the relay is turned on for 5 seconds and then turned off. The current data is also sent to the serial monitor for monitoring purposes.
Controlling the Intelligent Power Switch remotely
To control the Intelligent Power Switch remotely, you can add a communication interface to the microcontroller, such as Wi-Fi or Bluetooth. Here’s an example of how to control the Intelligent Power Switch using an ESP32 microcontroller and Wi-Fi:
- Connect the ESP32 to the Intelligent Power Switch circuit.
- Program the ESP32 to create a web server that accepts commands to turn the power on or off.
- Connect the ESP32 to a Wi-Fi network.
- Access the web server using a browser or send HTTP requests to control the Intelligent Power Switch remotely.
Example ESP32 code for remote control:
#include <WiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const int relayPin = 2;
WiFiServer server(80);
void setup() {
pinMode(relayPin, OUTPUT);
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("WiFi connected");
Serial.println(WiFi.localIP());
server.begin();
}
void loop() {
WiFiClient client = server.available();
if (client) {
String request = client.readStringUntil('\r');
Serial.println(request);
client.flush();
if (request.indexOf("/ON") != -1) {
digitalWrite(relayPin, HIGH);
} else if (request.indexOf("/OFF") != -1) {
digitalWrite(relayPin, LOW);
}
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("");
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<h1>Intelligent Power Switch Control</h1>");
client.println("<p><a href=\"/ON\"><button>ON</button></a></p>");
client.println("<p><a href=\"/OFF\"><button>OFF</button></a></p>");
client.println("</html>");
}
}
This example code creates a web server on the ESP32 that allows users to control the Intelligent Power Switch by clicking on “ON” and “OFF” buttons on a web page served by the ESP32. The ESP32 connects to a Wi-Fi network and listens for incoming HTTP requests. When a request is received, it checks if the request contains “/ON” or “/OFF” and sets the relay state accordingly.
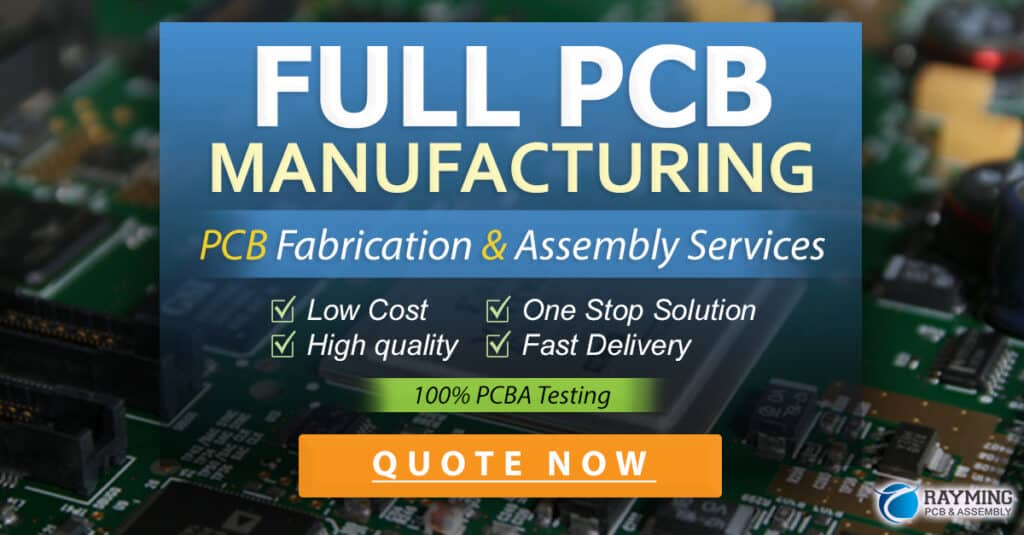
Applications of Intelligent Power Switches with Raspberry Pi
Home automation
Intelligent Power Switches can be used in home automation projects to control various devices connected to a Raspberry Pi, such as lights, fans, or appliances. By integrating the Intelligent Power Switch with sensors and a home automation platform like Home Assistant or OpenHAB, users can create automated power management scenarios based on factors like time, presence, or environmental conditions.
Example scenario: Turn on a connected lamp when motion is detected in a room and turn it off after a specified period of inactivity.
Remote monitoring and control of Raspberry Pi-based systems
In remote monitoring and control applications, Intelligent Power Switches enable users to manage the power state of Raspberry Pi-based systems from anywhere with an internet connection. This is particularly useful for systems deployed in remote locations or for managing multiple Raspberry Pi devices in a distributed network.
Example scenario: Remotely reboot a Raspberry Pi-based weather station if it becomes unresponsive or fails to send data.
Energy optimization in Raspberry Pi clusters
Raspberry Pi clusters, used for parallel computing or distributed tasks, can benefit from Intelligent Power Switches to optimize energy consumption. By monitoring the power usage of individual Raspberry Pi nodes and controlling their power state based on workload or idle time, users can reduce overall energy consumption and extend the lifespan of the devices.
Example scenario: Turn off idle Raspberry Pi nodes in a cluster when they are not processing tasks and turn them back on when needed.
Conclusion
Intelligent Power Switches are valuable tools for optimizing power management, protecting devices, and enabling remote control in Raspberry Pi-based projects. By integrating these switches with sensors, microcontrollers, and communication interfaces, users can create automated power management scenarios and remotely control the power state of their Raspberry Pi devices. As Raspberry Pi continues to be a popular choice for various applications, Intelligent Power Switches will play an increasingly important role in ensuring efficient and reliable operation of these systems.
Frequently Asked Questions (FAQ)
-
Can an Intelligent Power Switch be used with other single-board computers besides Raspberry Pi?
Yes, Intelligent Power Switches can be used with other single-board computers that have similar power requirements and connectivity options, such as Beaglebone, ODROID, or NVIDIA Jetson. -
What is the maximum power rating for an Intelligent Power Switch?
The maximum power rating for an Intelligent Power Switch depends on the specific components used, such as the relay or MOSFET. Common relays can handle up to 10A or 250VAC, while high-power relays can switch higher currents. It is essential to choose components that match the power requirements of the connected devices. -
Can multiple devices be controlled with a single Intelligent Power Switch?
Yes, multiple devices can be controlled with a single Intelligent Power Switch by using a relay module with multiple channels or by connecting multiple relays to the microcontroller. Each device would be connected to a separate relay channel, allowing independent control of each device. -
Is it possible to integrate an Intelligent Power Switch with solar power systems?
Yes, Intelligent Power Switches can be integrated with solar power systems to optimize energy usage and manage power distribution. The switch can monitor the solar power generation and battery levels, and control the connected devices based on the available power or predefined priorities. -
Are there any pre-built Intelligent Power Switch solutions available for Raspberry Pi?
There are some pre-built Intelligent Power Switch solutions available for Raspberry Pi, such as the PiJuice HAT or the Witty Pi 3. These solutions offer features like battery backup, real-time clock, and power management software, making it easier to implement intelligent power control in Raspberry Pi projects. However, building a custom Intelligent Power Switch allows for greater flexibility and customization based on specific project requirements.
Leave a Reply