Table of Contents
- What is a React Component?
- Class Components
- Syntax and Structure
- Lifecycle Methods
- State Management
- Event Handling
- Functional Components
- Syntax and Structure
- Props
- State Management with Hooks
- Performance and Simplicity
- Choosing Between Class and Functional Components
- Frequently Asked Questions (FAQ)
- Conclusion
What is a React Component?
Before diving into the specifics of class and functional components, let’s briefly review what a React component is. A component in React is a self-contained piece of code that represents a part of the user interface. It can be reused throughout the application and can receive data through props (short for properties).
Components are the building blocks of a React application. They allow you to break down the UI into smaller, reusable pieces, making your code more modular and easier to maintain. Components can be nested inside other components to create complex UIs.
Class Components
Class components are defined using ES6 class syntax and extend the React.Component
base class. They provide a more feature-rich way of creating components compared to functional components.
Syntax and Structure
Here’s an example of a basic class component:
import React from 'react';
class MyComponent extends React.Component {
render() {
return <div>Hello, {this.props.name}!</div>;
}
}
export default MyComponent;
In this example, MyComponent
is a class component that extends React.Component
. It has a render()
method that returns JSX, which defines the structure and appearance of the component. The component receives data through this.props
, which allows it to be dynamic based on the parent component.
Lifecycle Methods
One of the key features of class components is their lifecycle methods. Lifecycle methods are special methods that allow you to hook into different stages of a component’s life cycle and perform actions at specific points.
Here are some commonly used lifecycle methods:
constructor(props)
: Called when the component is initialized. It is used to set the initial state and bind event handlers.componentDidMount()
: Called after the component is mounted to the DOM. It is used for performing side effects, such as fetching data from an API.componentDidUpdate(prevProps, prevState)
: Called after the component updates. It is used for performing side effects based on changes in props or state.componentWillUnmount()
: Called before the component is unmounted and destroyed. It is used for cleanup, such as canceling timers or subscriptions.
These lifecycle methods provide granular control over the component’s behavior and allow you to execute code at specific points in the component’s life cycle.
State Management
Class components have their own internal state, which can be managed using the state
property. State represents the mutable data that the component owns and can change over time.
To initialize the state, you can set it in the constructor()
:
constructor(props) {
super(props);
this.state = {
count: 0
};
}
To update the state, you should use the setState()
method:
incrementCount() {
this.setState((prevState) => ({
count: prevState.count + 1
}));
}
Whenever the state is updated using setState()
, React will re-render the component and its child components to reflect the new state.
Event Handling
Class components can handle events by defining event handler methods. These methods are typically arrow functions to automatically bind this
to the component instance.
Here’s an example of handling a button click event:
handleClick = () => {
console.log('Button clicked!');
};
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
In this example, the handleClick
method is defined as an arrow function and is passed as the onClick
event handler to the button. When the button is clicked, the handleClick
method will be invoked.
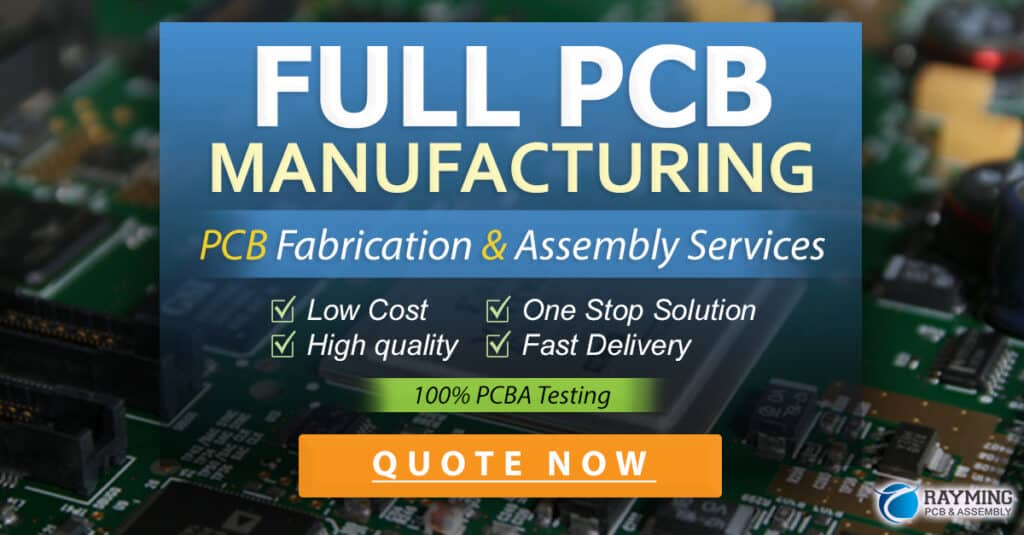
Functional Components
Functional components, also known as stateless components or presentational components, are simpler and more lightweight compared to class components. They are defined as JavaScript functions that take props as input and return JSX.
Syntax and Structure
Here’s an example of a basic functional component:
import React from 'react';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
export default MyComponent;
In this example, MyComponent
is a functional component that receives props
as a parameter. It returns JSX that represents the structure and appearance of the component.
Props
Functional components receive data through props
, which are passed down from the parent component. Props are read-only and cannot be modified by the component itself.
Here’s an example of using props in a functional component:
function Greeting(props) {
return <div>Hello, {props.name}! Your age is {props.age}.</div>;
}
In this example, the Greeting
component receives name
and age
as props and uses them to render a personalized greeting.
State Management with Hooks
With the introduction of React Hooks, functional components gained the ability to manage state and perform side effects. Hooks allow you to add state and other React features to functional components without the need for class components.
The most commonly used hook for state management is the useState
hook. It allows you to declare state variables and provides a way to update them.
Here’s an example of using the useState
hook in a functional component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
In this example, the Counter
component uses the useState
hook to manage the count
state variable. The setCount
function is used to update the state when the increment button is clicked.
Performance and Simplicity
Functional components have a simpler syntax and are generally more concise compared to class components. They are easier to understand and test since they are plain JavaScript functions.
Functional components also have a performance advantage because they don’t have the overhead of a class instance and lifecycle methods. They are lightweight and can result in faster rendering times.
Choosing Between Class and Functional Components
When deciding whether to use a class component or a functional component, consider the following guidelines:
- If the component needs to manage state or use lifecycle methods, use a class component.
- If the component is simple and only needs to receive props and render JSX, use a functional component.
- With the introduction of hooks, functional components can now manage state and perform side effects, making them more versatile.
- If you are comfortable with hooks and don’t need the specific features of class components, you can use functional components for most cases.
Here’s a comparison table to help you choose between class and functional components:
Feature | Class Component | Functional Component |
---|---|---|
State Management | Yes | Yes (with hooks) |
Lifecycle Methods | Yes | No |
Performance | Slightly slower | Faster |
Syntax | More complex | Simpler |
Reusability | Yes | Yes |
Testing | More setup | Easier to test |
Ultimately, the choice between class and functional components depends on your specific requirements and the complexity of your component. Both types of components have their strengths and can be used effectively in a React application.
Frequently Asked Questions (FAQ)
-
Can functional components replace class components entirely?
With the introduction of hooks, functional components have become more powerful and can handle most use cases previously requiring class components. However, there may still be situations where class components are preferred, such as when using certain third-party libraries or legacy code. -
Are class components going to be deprecated in the future?
There are no plans to deprecate class components in React. They will continue to be supported, and existing codebases using class components will still work. However, the React community has largely embraced functional components and hooks as the preferred way of writing components. -
Can I mix class and functional components in the same application?
Yes, you can mix class and functional components in the same React application. They can coexist and work together seamlessly. You can even have a class component render a functional component and vice versa. -
How do I share state between components?
To share state between components, you can lift the state up to a common parent component and pass it down as props to the child components that need access to it. Alternatively, you can use state management libraries like Redux or the Context API to manage shared state globally. -
What are some best practices for writing components in React?
Some best practices for writing components in React include: - Keep components small and focused on a single responsibility.
- Use meaningful and descriptive names for components and props.
- Avoid deep nesting of components and keep the component tree flat.
- Use functional components and hooks whenever possible for simplicity and performance.
- Follow a consistent code style and naming convention throughout your application.
Conclusion
Class components and functional components are the two main types of components in React. Class components provide a more feature-rich approach with lifecycle methods and state management, while functional components offer simplicity and performance benefits.
With the introduction of hooks, functional components have become more powerful and can handle most use cases previously requiring class components. However, class components still have their place and can be used when specific features or integrations are needed.
When deciding between class and functional components, consider the complexity of your component, the need for state management and lifecycle methods, and your personal preference and familiarity with hooks.
Regardless of the component type you choose, writing clean, modular, and reusable components is key to building maintainable and scalable React applications. By understanding the differences and use cases of class and functional components, you can make informed decisions and create high-quality user interfaces.
Leave a Reply