Introduction to the DB31 Altera Cyclone II Daughter Board
The DB31 Altera Cyclone II Daughter Board is a versatile and powerful development board designed for rapid prototyping and implementation of digital logic circuits. Based on the Altera Cyclone II FPGA (Field Programmable Gate Array), this board offers a wide range of features and capabilities that make it an ideal choice for both beginners and experienced designers.
Key Features of the DB31 Altera Cyclone II Daughter Board
- Altera Cyclone II EP2C20F484C7 FPGA
- 32MB SDRAM
- 2MB Flash memory
- 4 user LEDs
- 4 user push buttons
- 50MHz oscillator
- USB Blaster interface for programming and debugging
- Expansion headers for additional peripherals
The Altera Cyclone II FPGA is the heart of the DB31 daughter board, providing a flexible and reconfigurable platform for implementing digital logic designs. With 20,060 logic elements and 294,912 bits of embedded memory, the Cyclone II FPGA offers ample resources for a wide range of applications, from simple combinatorial circuits to complex sequential designs.
Getting Started with the DB31 Altera Cyclone II Daughter Board
Hardware Setup
To begin using the DB31 Altera Cyclone II Daughter Board, follow these steps:
- Ensure that your host computer has the necessary software installed, including the Altera Quartus II development environment and the USB Blaster driver.
- Connect the DB31 daughter board to your host computer using a USB cable. The board will be powered through the USB connection.
- Launch the Altera Quartus II software and create a new project.
- Configure the project settings to target the Cyclone II EP2C20F484C7 FPGA.
- Design your digital logic circuit using the Quartus II tools, such as the schematic editor or the hardware description language (HDL) editor.
- Compile your design and generate the programming file.
- Use the USB Blaster interface to download the programming file to the DB31 daughter board.
- Test and debug your design using the on-board LEDs, push buttons, and other peripherals.
Software Development
The Altera Quartus II development environment provides a comprehensive set of tools for designing, simulating, and implementing digital logic circuits on the DB31 Altera Cyclone II Daughter Board. The software includes:
- Schematic editor for graphical design entry
- Hardware description language (HDL) editor for Verilog and VHDL design entry
- Functional and timing simulation tools
- Logic synthesis and optimization tools
- Place and route tools for FPGA implementation
- Debugging and analysis tools
To create a new project in Quartus II, follow these steps:
- Launch the Quartus II software and select “File” > “New Project Wizard”.
- Specify the project name and location, then click “Next”.
- Select the target device family (Cyclone II) and the specific device (EP2C20F484C7), then click “Next”.
- Choose the project template (empty project or a specific template), then click “Next”.
- Review the project summary and click “Finish”.
Once your project is created, you can begin designing your digital logic circuit using the schematic editor or the HDL editor. The Quartus II software provides a wide range of design entry, synthesis, and implementation tools to help you create efficient and reliable designs.
Example Designs for the DB31 Altera Cyclone II Daughter Board
Simple LED Blink Circuit
One of the most basic examples of a digital logic circuit is a simple LED blink circuit. This design toggles an LED on and off at a fixed interval, demonstrating the basic functionality of the DB31 Altera Cyclone II Daughter Board.
Here is an example Verilog code for a simple LED blink circuit:
module led_blink (
input wire clk,
output reg led
);
parameter COUNTER_WIDTH = 26;
reg [COUNTER_WIDTH-1:0] counter;
always @(posedge clk) begin
if (counter == 0) begin
led <= ~led;
counter <= (2 ** COUNTER_WIDTH) - 1;
end else begin
counter <= counter - 1;
end
end
endmodule
In this example, the led_blink
module uses a 26-bit counter to divide the input clock frequency and toggle the LED state when the counter reaches zero. The LED blink rate can be adjusted by modifying the COUNTER_WIDTH
parameter.
7-Segment Display Counter
Another common example design is a 7-segment display counter, which increments a count value and displays it on a 7-segment LED display. This design demonstrates the use of the DB31 daughter board’s expansion headers to interface with external peripherals.
Here is an example Verilog code for a 7-segment display counter:
module seven_seg_counter (
input wire clk,
input wire rst_n,
output reg [6:0] seg,
output reg [3:0] an
);
parameter COUNTER_WIDTH = 26;
reg [COUNTER_WIDTH-1:0] counter;
reg [3:0] count;
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
counter <= 0;
count <= 0;
end else if (counter == 0) begin
counter <= (2 ** COUNTER_WIDTH) - 1;
count <= count + 1;
end else begin
counter <= counter - 1;
end
end
always @(*) begin
case (count)
4'h0: seg = 7'b1000000;
4'h1: seg = 7'b1111001;
4'h2: seg = 7'b0100100;
4'h3: seg = 7'b0110000;
4'h4: seg = 7'b0011001;
4'h5: seg = 7'b0010010;
4'h6: seg = 7'b0000010;
4'h7: seg = 7'b1111000;
4'h8: seg = 7'b0000000;
4'h9: seg = 7'b0010000;
default: seg = 7'b1111111;
endcase
an = 4'b1110;
end
endmodule
In this example, the seven_seg_counter
module uses a 26-bit counter to divide the input clock frequency and increment a 4-bit count value. The count value is then decoded and displayed on a 7-segment LED display connected to the DB31 daughter board’s expansion headers. The an
signal is used to select the active digit on the display.
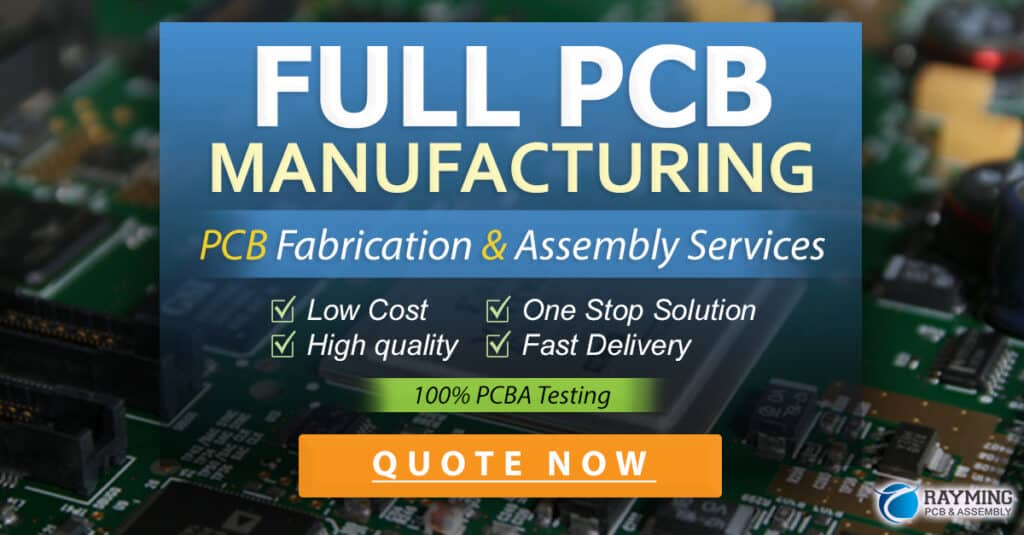
Interfacing with External Peripherals
The DB31 Altera Cyclone II Daughter Board provides expansion headers that allow you to connect external peripherals and extend the functionality of your designs. Some common peripherals that can be interfaced with the DB31 daughter board include:
- Sensors (e.g., temperature, pressure, light)
- Actuators (e.g., motors, servos)
- Communication modules (e.g., UART, SPI, I2C)
- Memory devices (e.g., external SRAM, EEPROM)
- Display modules (e.g., LCD, OLED)
To interface with an external peripheral, you will need to:
- Identify the appropriate expansion header pins for the peripheral’s signals.
- Design the necessary digital logic circuits to communicate with the peripheral using the appropriate protocol (e.g., UART, SPI, I2C).
- Implement the interface logic in your Quartus II project.
- Connect the peripheral to the DB31 daughter board’s expansion headers.
- Test and debug the interface using the Quartus II software and the on-board debugging tools.
Here is an example of interfacing with an external UART module using the DB31 Altera Cyclone II Daughter Board:
module uart_interface (
input wire clk,
input wire rst_n,
input wire rx,
output wire tx,
output reg [7:0] data_out,
output reg data_valid
);
parameter BAUD_RATE = 9600;
parameter CLK_FREQ = 50_000_000;
localparam BAUD_COUNTER_MAX = CLK_FREQ / BAUD_RATE;
reg [7:0] tx_data;
reg tx_start;
wire tx_busy;
reg [3:0] rx_state;
reg [3:0] tx_state;
reg [15:0] baud_counter;
reg [2:0] bit_counter;
// UART receiver
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
rx_state <= 0;
baud_counter <= 0;
bit_counter <= 0;
data_out <= 0;
data_valid <= 0;
end else begin
case (rx_state)
// Idle state
0: begin
if (!rx) begin
rx_state <= 1;
baud_counter <= BAUD_COUNTER_MAX / 2;
end
end
// Start bit
1: begin
if (baud_counter == 0) begin
rx_state <= 2;
baud_counter <= BAUD_COUNTER_MAX;
bit_counter <= 0;
end else begin
baud_counter <= baud_counter - 1;
end
end
// Data bits
2: begin
if (baud_counter == 0) begin
data_out[bit_counter] <= rx;
bit_counter <= bit_counter + 1;
if (bit_counter == 7) begin
rx_state <= 3;
end
baud_counter <= BAUD_COUNTER_MAX;
end else begin
baud_counter <= baud_counter - 1;
end
end
// Stop bit
3: begin
if (baud_counter == 0) begin
data_valid <= 1;
rx_state <= 0;
end else begin
baud_counter <= baud_counter - 1;
end
end
endcase
end
end
// UART transmitter
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
tx_state <= 0;
baud_counter <= 0;
bit_counter <= 0;
tx <= 1;
end else begin
case (tx_state)
// Idle state
0: begin
if (tx_start) begin
tx_state <= 1;
baud_counter <= BAUD_COUNTER_MAX;
tx <= 0;
end
end
// Start bit
1: begin
if (baud_counter == 0) begin
tx_state <= 2;
baud_counter <= BAUD_COUNTER_MAX;
bit_counter <= 0;
end else begin
baud_counter <= baud_counter - 1;
end
end
// Data bits
2: begin
if (baud_counter == 0) begin
tx <= tx_data[bit_counter];
bit_counter <= bit_counter + 1;
if (bit_counter == 7) begin
tx_state <= 3;
end
baud_counter <= BAUD_COUNTER_MAX;
end else begin
baud_counter <= baud_counter - 1;
end
end
// Stop bit
3: begin
if (baud_counter == 0) begin
tx <= 1;
tx_state <= 0;
end else begin
baud_counter <= baud_counter - 1;
end
end
endcase
end
end
assign tx_busy = (tx_state != 0);
endmodule
In this example, the uart_interface
module implements a simple UART receiver and transmitter. The receiver samples the incoming data on the rx
pin and outputs the received byte on the data_out
port when a complete byte has been received. The transmitter sends data on the tx
pin when the tx_start
signal is asserted and the tx_data
input contains the byte to be transmitted. The tx_busy
signal indicates when the transmitter is actively sending data.
To use this UART interface with the DB31 daughter board, you would need to:
- Connect the UART module’s
rx
andtx
pins to the appropriate expansion header pins on the DB31 daughter board. - Instantiate the
uart_interface
module in your top-level design. - Provide the necessary clock and reset signals to the
uart_interface
module. - Assert the
tx_start
signal and provide thetx_data
byte when you want to transmit data. - Monitor the
data_valid
signal and read thedata_out
byte when a complete byte has been received.
Debugging and Testing
The DB31 Altera Cyclone II Daughter Board provides several features that facilitate debugging and testing your digital logic designs. These include:
- On-board LEDs and push buttons for simple input/output testing
- USB Blaster interface for programming and debugging
- Expansion headers for connecting logic analyzers or other debugging tools
To debug your design using the USB Blaster interface and the Quartus II software, follow these steps:
- Ensure that your design is compiled and the programming file is generated.
- Connect the DB31 daughter board to your host computer using a USB cable.
- Launch the Quartus II Programmer and select the appropriate programming file.
- Configure the programming options and select the USB Blaster as the programming hardware.
- Click “Start” to download the programming file to the DB31 daughter board.
- Use the Quartus II SignalTap Logic Analyzer or other debugging tools to monitor internal signals and diagnose issues in your design.
For more advanced debugging, you can use external logic analyzers or oscilloscopes connected to the DB31 daughter board’s expansion headers. This allows you to capture and analyze signals that may not be accessible through the USB Blaster interface.
When testing your design, it is important to develop a comprehensive test plan that covers all the key functionalities and edge cases. This may include:
- Basic input/output tests using the on-board LEDs and push buttons
- Functional tests for each module or subsystem in your design
- Integration tests to verify the interaction between different modules
- Boundary condition tests to ensure proper handling of extreme or unexpected inputs
- Performance tests to measure the speed and resource utilization of your design
By thoroughly testing your design, you can identify and correct issues early in the development process, reducing the risk of failures or unexpected behavior in the final implementation.
Advanced Topics and Resources
As you become more experienced with the DB31 Altera Cyclone II Daughter Board and digital logic design, you may want to explore more advanced topics and resources. Some areas of interest include:
- High-level synthesis (HLS) for accelerating algorithm development
- Embedded processor design using the Nios II soft processor
- Digital signal processing (DSP) applications
- Networking and communication protocols
- System-on-chip (SoC) design and integration
Here are some resources to help you further your knowledge and skills:
- Altera Cyclone II Device Handbook: https://www.intel.com/content/www/us/en/programmable/documentation/sam1403479399088.html
- Altera Quartus II Handbook: https://www.intel.com/content/www/us/en/programmable/documentation/mwh1409960181641.html
- Verilog HDL and SystemVerilog resources:
- “Verilog HDL: A Guide to Digital Design and Synthesis” by Samir Palnitkar
- “SystemVerilog for Design” by Stuart Sutherland, Simon Davidmann, and Peter Flake
- FPGA design and implementation resources:
- “FPGA Prototyping by Verilog Examples” by Pong P. Chu
- “The Designer’s Guide to VHDL” by Peter J. Ashenden
5.
Leave a Reply