Introduction to ConstraintsManager DLG ScopesLibraryScopes
ConstraintsManager DLG ScopesLibraryScopes is a powerful library that provides a comprehensive set of tools for managing constraints and scopes in software development. It is designed to simplify the process of defining, organizing, and applying constraints to various aspects of a software system, such as database queries, user interface components, and business logic.
The library offers a wide range of features that enable developers to create robust and maintainable applications by enforcing consistency, reducing errors, and improving code readability. Some of the key features of ConstraintsManager DLG ScopesLibraryScopes include:
- Declarative constraint definition
- Scope-based constraint organization
- Flexible constraint application
- Extensible architecture
- Integration with popular frameworks and libraries
In this article, we will explore the ConstraintsManager DLG ScopesLibraryScopes library in detail, covering its core concepts, usage patterns, and best practices. We will also provide examples and code snippets to illustrate how the library can be used in real-world scenarios.
Understanding Constraints and Scopes
What are Constraints?
Constraints are rules or conditions that must be satisfied by a software system or its components. They help ensure the integrity, consistency, and correctness of data and behavior within an application. Constraints can be applied at various levels, such as:
- Database level: Ensuring data integrity and consistency
- User interface level: Validating user input and enforcing business rules
- Business logic level: Enforcing domain-specific rules and regulations
Some common examples of constraints include:
- Data type constraints: Ensuring that a value is of a specific data type (e.g., string, integer, date)
- Range constraints: Ensuring that a value falls within a specified range (e.g., age between 18 and 65)
- Required field constraints: Ensuring that a value is provided for a mandatory field
- Unique key constraints: Ensuring that a value is unique within a specific context (e.g., email address)
What are Scopes?
Scopes are logical boundaries within which constraints are defined and applied. They provide a way to organize and manage constraints based on their applicability and context. Scopes can be hierarchical, allowing for the inheritance and overriding of constraints at different levels.
Some common examples of scopes include:
- Application scope: Constraints that apply to the entire application
- Module scope: Constraints that apply to a specific module or component of the application
- Entity scope: Constraints that apply to a specific entity or data model
- Field scope: Constraints that apply to a specific field or property of an entity
By organizing constraints into scopes, developers can achieve better code organization, reusability, and maintainability. Scopes also enable the selective application of constraints based on the specific requirements of different parts of the application.
ConstraintsManager DLG ScopesLibraryScopes Architecture
The ConstraintsManager DLG ScopesLibraryScopes library follows a modular and extensible architecture that allows for easy integration and customization. The key components of the library include:
- Constraint Definition API
- Scope Management API
- Constraint Validation API
- Constraint Metadata API
- Integration Adapters
Constraint Definition API
The Constraint Definition API provides a declarative way to define constraints using a fluent and expressive syntax. It allows developers to specify constraints using a combination of built-in and custom constraint types, along with their associated parameters and error messages.
Here’s an example of defining a simple required field constraint using the Constraint Definition API:
var constraint = Constraint.Required()
.WithMessage("The {PropertyName} field is required.");
In this example, we define a required field constraint using the Required()
method and specify a custom error message using the WithMessage()
method. The {PropertyName}
placeholder will be replaced with the actual property name when the constraint is violated.
Scope Management API
The Scope Management API provides a way to define and manage scopes within the application. It allows developers to create scopes, associate constraints with scopes, and define scope hierarchies for inheritance and overriding of constraints.
Here’s an example of defining a scope and associating a constraint with it:
var scope = Scope.Create("Entity")
.AddConstraint(Constraint.Required()
.ForProperty("Name")
.WithMessage("The Name field is required."));
In this example, we create a scope named “Entity” using the Create()
method and add a required field constraint for the “Name” property using the AddConstraint()
method. The constraint is defined using the Constraint Definition API, specifying the property name and error message.
Constraint Validation API
The Constraint Validation API provides a way to validate objects against the defined constraints and scopes. It allows developers to execute validation checks and retrieve validation results, including any violated constraints and their associated error messages.
Here’s an example of validating an object against a scope:
var entity = new Entity { Name = null };
var validationResult = entity.Validate(scope);
if (!validationResult.IsValid)
{
foreach (var error in validationResult.Errors)
{
Console.WriteLine($"Property: {error.PropertyName}, Message: {error.ErrorMessage}");
}
}
In this example, we create an instance of the Entity
class with a null Name
property. We then validate the entity against the previously defined “Entity” scope using the Validate()
method. If the validation fails, we iterate over the validation errors and print the property name and error message for each violated constraint.
Constraint Metadata API
The Constraint Metadata API provides a way to retrieve metadata information about the defined constraints and scopes. It allows developers to programmatically inspect the constraint definitions, scope hierarchies, and associated metadata.
Here’s an example of retrieving metadata information about a scope:
var scopeMetadata = ScopeMetadata.Get("Entity");
Console.WriteLine($"Scope Name: {scopeMetadata.Name}");
Console.WriteLine("Constraints:");
foreach (var constraintMetadata in scopeMetadata.Constraints)
{
Console.WriteLine($"- {constraintMetadata.Type}: {constraintMetadata.PropertyName}");
}
In this example, we retrieve the metadata information for the “Entity” scope using the Get()
method of the ScopeMetadata
class. We then print the scope name and iterate over the associated constraint metadata, printing the constraint type and property name for each constraint.
Integration Adapters
The ConstraintsManager DLG ScopesLibraryScopes library provides integration adapters for popular frameworks and libraries, such as ASP.NET Core, Entity Framework, and AutoMapper. These adapters simplify the integration of the library into existing projects and enable seamless validation and mapping of constrained objects.
Here’s an example of using the ASP.NET Core integration adapter to automatically validate incoming request models:
public class UserController : ControllerBase
{
private readonly IUserService _userService;
public UserController(IUserService userService)
{
_userService = userService;
}
[HttpPost]
public IActionResult CreateUser([FromBody] CreateUserRequest request)
{
if (!ModelState.IsValid)
{
return BadRequest(ModelState);
}
var user = _userService.CreateUser(request);
return CreatedAtAction(nameof(GetUser), new { id = user.Id }, user);
}
// Other action methods...
}
In this example, we have a UserController
with a CreateUser
action method that accepts a CreateUserRequest
model. The ASP.NET Core integration adapter automatically validates the incoming request model against the defined constraints and populates the ModelState
with any validation errors. We can then check the ModelState.IsValid
property to determine if the request is valid and return an appropriate response.
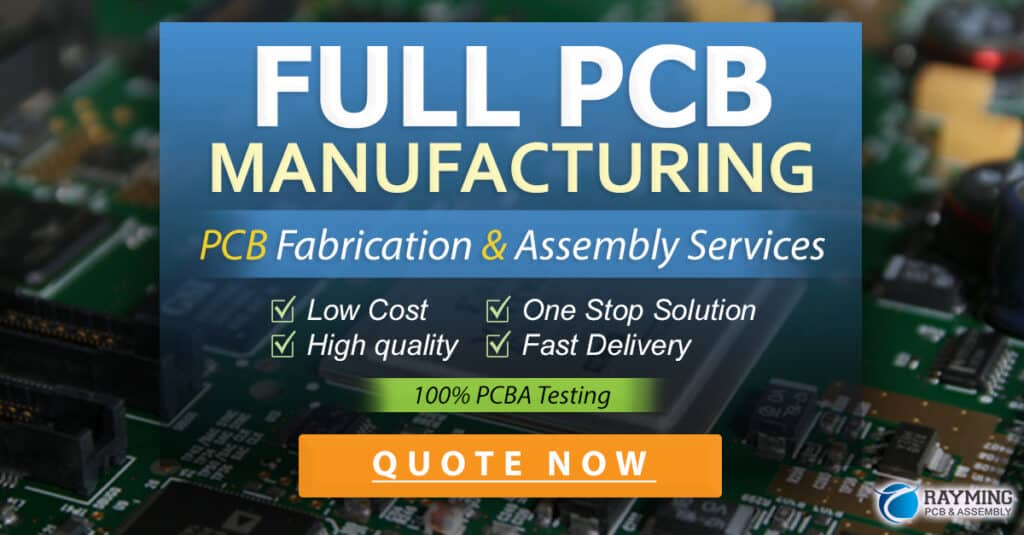
Best Practices and Guidelines
When using the ConstraintsManager DLG ScopesLibraryScopes library, there are several best practices and guidelines to follow to ensure maintainable and scalable code:
-
Define constraints in a centralized location: Keep all constraint definitions in a centralized location, such as a dedicated constraints folder or class, to improve code organization and maintainability.
-
Use meaningful constraint names and error messages: Use descriptive and meaningful names for constraints and provide clear and concise error messages to improve the user experience and facilitate debugging.
-
Leverage scope inheritance: Utilize scope inheritance to define common constraints at higher levels and override or extend them at lower levels as needed. This promotes code reuse and reduces duplication.
-
Validate early and often: Perform validation checks as early as possible in the application flow to catch and handle errors before they propagate further. Validate incoming data, business logic, and database operations to ensure data integrity and consistency.
-
Use appropriate constraint types: Choose the appropriate constraint types based on the specific requirements of your application. Use built-in constraint types when possible and create custom constraint types when needed to encapsulate complex validation logic.
-
Implement proper error handling: Handle validation errors gracefully by providing meaningful error messages to users and logging errors for debugging and monitoring purposes. Use appropriate HTTP status codes and response formats when building web APIs.
-
Keep constraints focused and modular: Keep constraints focused on a single responsibility and avoid mixing different types of constraints within the same scope or constraint definition. This promotes modularity and makes the code easier to understand and maintain.
-
Test constraints thoroughly: Write comprehensive unit tests to verify the behavior of constraints and ensure that they are applied correctly. Test different scenarios, including valid and invalid inputs, edge cases, and boundary conditions.
-
Document constraints and scopes: Provide clear and concise documentation for constraints and scopes, including their purpose, parameters, and usage guidelines. Use XML comments or API documentation tools to generate documentation automatically.
-
Monitor and audit constraint violations: Implement monitoring and auditing mechanisms to track constraint violations and identify potential issues in the application. Use logging frameworks or application performance monitoring tools to capture and analyze constraint violations.
By following these best practices and guidelines, you can effectively leverage the ConstraintsManager DLG ScopesLibraryScopes library to create robust, maintainable, and scalable applications.
Frequently Asked Questions (FAQ)
1. What is the purpose of the ConstraintsManager DLG ScopesLibraryScopes library?
The ConstraintsManager DLG ScopesLibraryScopes library is designed to simplify the process of defining, organizing, and applying constraints to various aspects of a software system. It provides a declarative way to define constraints, organize them into scopes, and validate objects against those constraints.
2. What are the key features of the ConstraintsManager DLG ScopesLibraryScopes library?
The key features of the ConstraintsManager DLG ScopesLibraryScopes library include:
– Declarative constraint definition using a fluent and expressive syntax
– Scope-based organization of constraints for better code organization and reusability
– Flexible constraint application and validation against objects
– Extensible architecture that allows for custom constraint types and integration with other frameworks
– Integration adapters for popular frameworks such as ASP.NET Core, Entity Framework, and AutoMapper
3. How do I define constraints using the ConstraintsManager DLG ScopesLibraryScopes library?
To define constraints using the ConstraintsManager DLG ScopesLibraryScopes library, you can use the Constraint Definition API. It provides a fluent and expressive syntax for specifying constraints, along with their associated parameters and error messages. For example:
var constraint = Constraint.Required()
.WithMessage("The {PropertyName} field is required.");
4. How do I organize constraints into scopes?
To organize constraints into scopes, you can use the Scope Management API provided by the ConstraintsManager DLG ScopesLibraryScopes library. You can create scopes, associate constraints with scopes, and define scope hierarchies for inheritance and overriding of constraints. For example:
var scope = Scope.Create("Entity")
.AddConstraint(Constraint.Required()
.ForProperty("Name")
.WithMessage("The Name field is required."));
5. How do I validate objects against constraints using the ConstraintsManager DLG ScopesLibraryScopes library?
To validate objects against constraints, you can use the Constraint Validation API provided by the ConstraintsManager DLG ScopesLibraryScopes library. You can execute validation checks on objects and retrieve validation results, including any violated constraints and their associated error messages. For example:
var entity = new Entity { Name = null };
var validationResult = entity.Validate(scope);
if (!validationResult.IsValid)
{
foreach (var error in validationResult.Errors)
{
Console.WriteLine($"Property: {error.PropertyName}, Message: {error.ErrorMessage}");
}
}
Conclusion
The ConstraintsManager DLG ScopesLibraryScopes library is a powerful tool for managing constraints and scopes in software development. It provides a declarative, expressive, and extensible way to define and apply constraints to various aspects of a software system.
By leveraging the library’s features, such as declarative constraint definition, scope-based organization, flexible validation, and integration with popular frameworks, developers can create robust, maintainable, and scalable applications. The library promotes code reusability, reduces errors, and improves code readability, leading to more efficient and effective software development.
To get the most out of the ConstraintsManager DLG ScopesLibraryScopes library, it is important to follow best practices and guidelines, such as defining constraints in a centralized location, using meaningful names and error messages, leveraging scope inheritance, validating early and often, and testing constraints thoroughly.
By incorporating the ConstraintsManager DLG ScopesLibraryScopes library into your software development workflow, you can streamline the process of managing constraints, ensure data integrity and consistency, and deliver high-quality applications that meet the evolving needs of your users.
Leave a Reply