What are Component Library Query Functions?
Component library query functions are a set of methods or APIs that enable developers to search for and retrieve components from a component library based on specific criteria. These functions allow developers to query the library using various parameters such as component name, type, properties, or even custom metadata. By providing a flexible and powerful querying mechanism, component library query functions make it easier for developers to find and utilize the components they need for their projects.
Benefits of Component Library Query Functions
-
Improved Efficiency: With component library query functions, developers can quickly locate the desired components without manually searching through the entire library. This saves valuable time and effort, allowing developers to focus on building the core functionality of their applications.
-
Enhanced Discoverability: Query functions enable developers to explore the available components in a library more effectively. By providing search capabilities based on various criteria, developers can discover components they may not have been aware of previously, leading to better utilization of the library’s resources.
-
Flexibility and Customization: Component library query functions offer flexibility in terms of the search parameters and criteria. Developers can query components based on specific properties, such as size, color, or behavior, allowing them to find components that precisely match their requirements. This level of customization helps in building more tailored and cohesive user interfaces.
-
Maintainability and Scalability: As component libraries grow in size and complexity, query functions become essential for maintaining and managing the library effectively. By providing a structured way to search and retrieve components, query functions make it easier to update, refactor, and scale the component library over time.
Implementing Component Library Query Functions
To implement component library query functions, several approaches can be taken depending on the specific requirements and technology stack of the project. Let’s explore some common techniques and considerations:
Query Language and Syntax
One of the key aspects of implementing query functions is defining a query language or syntax that developers can use to express their search criteria. The query language should be intuitive, expressive, and flexible enough to handle various search scenarios. Some popular query languages for component libraries include:
-
CSS Selectors: CSS selectors provide a familiar and widely-used syntax for querying elements based on their attributes, classes, or IDs. Many component libraries leverage CSS selectors as a query language, allowing developers to use selectors like
button[primary]
or.card.featured
to retrieve specific components. -
JSON-based Query Language: Some component libraries opt for a JSON-based query language, where developers can specify search criteria using a structured JSON object. This approach allows for more complex queries and can be easily serialized and deserialized for storage or transmission.
-
Custom Query Language: In some cases, component libraries may define their own custom query language tailored to their specific needs. This allows for more fine-grained control over the querying process and can provide additional features or optimizations specific to the library.
Query Execution and Optimization
Once the query language is defined, the next step is to implement the actual query execution logic. This involves parsing the query, traversing the component library’s data structure, and filtering the components based on the specified criteria. To ensure efficient query execution, several optimization techniques can be employed:
-
Indexing: Building indexes on frequently queried properties or attributes can significantly speed up the query process. By creating indexes, the library can avoid scanning the entire component collection and instead quickly locate the relevant components based on the indexed values.
-
Caching: Caching the results of frequently executed queries can help reduce the overhead of repeated queries. By storing the query results in memory or a cache storage, subsequent queries with the same criteria can be served from the cache, avoiding the need to re-execute the query.
-
Lazy Loading: In large component libraries, loading all components upfront can be resource-intensive and impact performance. Lazy loading techniques can be used to load components on-demand based on the query results. This approach ensures that only the necessary components are loaded into memory, improving the overall efficiency of the library.
Integration with Development Workflow
To maximize the benefits of component library query functions, it is important to integrate them seamlessly into the development workflow. This involves providing developer-friendly APIs, documentation, and tooling to facilitate the usage of query functions. Some considerations for integration include:
-
API Design: The query function API should be intuitive, well-documented, and follow best practices for naming conventions and parameter handling. It should provide clear and concise methods for executing queries and retrieving the results.
-
Documentation and Examples: Comprehensive documentation should be provided to guide developers on how to use the query functions effectively. Code examples, tutorials, and use cases can help developers understand the capabilities and usage patterns of the query functions.
-
IDE Integration: Integrating query functions with popular Integrated Development Environments (IDEs) can greatly enhance the developer experience. This can include features like auto-completion, syntax highlighting, and contextual documentation for the query language and API.
-
Testing and Validation: Implementing robust testing and validation mechanisms for query functions is crucial to ensure their reliability and correctness. Unit tests, integration tests, and query validation can help catch potential issues and maintain the integrity of the query results.
Best Practices for Effective Query Functions
To create effective and user-friendly query functions for component libraries, consider the following best practices:
-
Keep It Simple: Strive for simplicity in the query language and API design. A simple and intuitive query syntax will make it easier for developers to understand and use the query functions effectively.
-
Provide Meaningful Error Messages: When queries fail or return unexpected results, provide clear and informative error messages. This helps developers identify and resolve issues quickly, improving the overall development experience.
-
Optimize for Performance: Ensure that the query execution is optimized for performance, especially for large component libraries. Employ techniques like indexing, caching, and lazy loading to minimize the query response time and resource consumption.
-
Support Common Use Cases: Identify and support the most common query use cases and patterns. This may include querying components by type, name, properties, or custom metadata. By addressing the typical query scenarios, you can cater to the needs of the majority of developers.
-
Maintain Consistency: Ensure consistency in the query language, API design, and behavior across different parts of the component library. Consistent naming conventions, parameter handling, and result formatting make it easier for developers to work with the query functions.
-
Provide Versioning and Backward Compatibility: As the component library evolves, it is important to maintain backward compatibility for query functions. Use versioning techniques to introduce new features or changes without breaking existing queries and ensure a smooth transition for developers.
-
Gather Feedback and Iterate: Continuously gather feedback from developers using the component library and its query functions. Collect insights on usability, performance, and feature requests. Iterate and improve the query functions based on the feedback to meet the evolving needs of the development community.
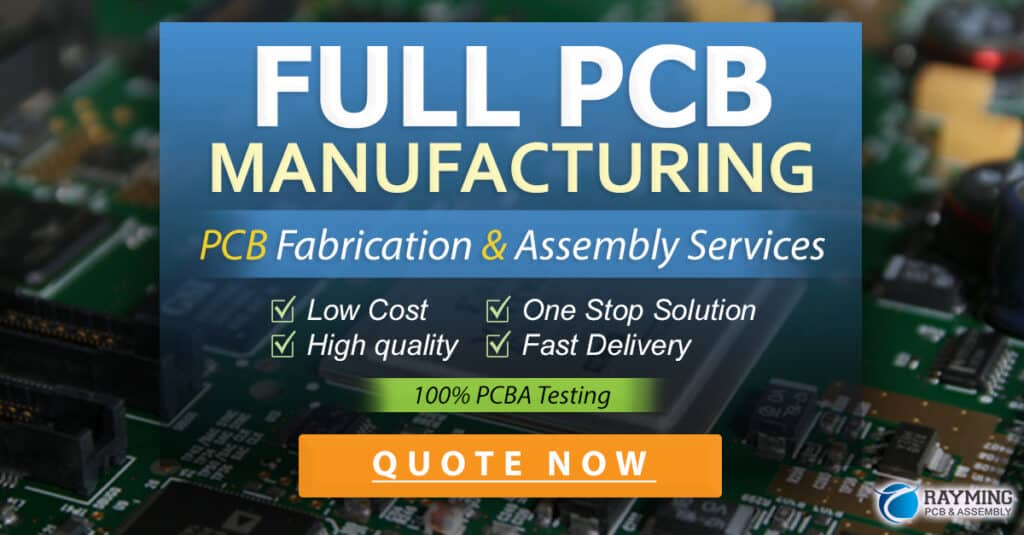
Real-World Examples
To better understand the practical application of component library query functions, let’s explore a few real-world examples:
Example 1: Material-UI
Material-UI is a popular React component library that provides a wide range of pre-built UI components following the Material Design guidelines. Material-UI offers a powerful styling solution called “CSS-in-JS” using the styled
function. This function allows developers to query and style components based on their properties.
import { styled } from '@material-ui/core/styles';
const PrimaryButton = styled(Button)({
backgroundColor: 'primary.main',
color: 'white',
'&:hover': {
backgroundColor: 'primary.dark',
},
});
In this example, the styled
function is used to create a custom PrimaryButton
component by querying and styling the base Button
component. The query criteria are specified using an object syntax, where properties like backgroundColor
and color
are set. The &:hover
syntax allows querying and styling the button’s hover state.
Example 2: Storybook
Storybook is a popular tool for developing and documenting UI components in isolation. It provides a way to organize and showcase components in a component library. Storybook supports querying components using a simple format called “Component Story Format” (CSF).
import { Button } from './Button';
export default {
title: 'Components/Button',
component: Button,
};
export const Primary = () => <Button primary>Primary</Button>;
export const Secondary = () => <Button secondary>Secondary</Button>;
In this example, the Button
component is imported and defined as the default export with a title and component property. The Primary
and Secondary
named exports represent different stories or variations of the button component. These stories can be queried and rendered in Storybook’s UI for documentation and testing purposes.
Example 3: Vuetify
Vuetify is a comprehensive Vue.js component library that provides a set of pre-built UI components following the Material Design specification. Vuetify uses a declarative approach for querying and configuring components through props and attributes.
<template>
<v-btn color="primary" outlined>
Primary Button
</v-btn>
</template>
<script>
import { VBtn } from 'vuetify/lib';
export default {
components: {
VBtn,
},
};
</script>
In this example, the v-btn
component is used to render a button. The color
and outlined
props are used to query and configure the button’s appearance. Vuetify’s query syntax allows developers to easily customize components using declarative attributes directly in the template.
These examples demonstrate how different component libraries and tools leverage query functions to provide flexible and efficient ways to work with components.
Frequently Asked Questions (FAQ)
-
What are the benefits of using component library query functions?
Component library query functions offer several benefits, including improved efficiency in locating components, enhanced discoverability of available components, flexibility in customizing search criteria, and better maintainability and scalability of the component library. -
How do I choose the right query language for my component library?
The choice of query language depends on factors such as the complexity of your component library, the familiarity of your development team with existing query languages, and the specific requirements of your project. Popular options include CSS selectors, JSON-based query languages, or custom query languages tailored to your library’s needs. -
What performance considerations should I keep in mind when implementing query functions?
To ensure efficient query execution, consider techniques like indexing frequently queried properties, caching query results, and lazy loading components. These optimizations can significantly improve the performance of your component library, especially for large-scale projects. -
How can I integrate query functions into my development workflow?
To integrate query functions seamlessly into your development workflow, provide well-documented APIs, comprehensive documentation with examples, and consider integrating with popular IDEs for auto-completion and syntax highlighting. Additionally, implement robust testing and validation mechanisms to ensure the reliability and correctness of query results. -
What are some best practices for creating effective and user-friendly query functions?
Some best practices include keeping the query language simple and intuitive, providing meaningful error messages, optimizing for performance, supporting common use cases, maintaining consistency across the library, ensuring backward compatibility, and gathering feedback from developers to iterate and improve the query functions over time.
Conclusion
Component library query functions play a crucial role in enhancing the usability and productivity of component libraries. By providing flexible and powerful querying capabilities, developers can easily search for and retrieve specific components based on their properties and attributes. Implementing effective query functions involves defining a suitable query language, optimizing query execution, integrating with development workflows, and following best practices for usability and maintainability.
As component libraries continue to evolve and grow in complexity, the importance of query functions will only increase. By investing in robust and user-friendly query functions, component library authors can provide developers with the tools they need to build efficient and maintainable applications. With the right approach and best practices, component library query functions can significantly streamline the development process and empower developers to create exceptional user experiences.
Leave a Reply