What is Via Style?
Via style refers to the visual styling and appearance of elements in a user interface or webpage. It encompasses properties such as colors, fonts, spacing, borders, and other visual attributes that define how elements are presented to the user.
In the context of web development, via style is often controlled using CSS (Cascading Style Sheets). CSS allows developers to separate the presentation and styling of a webpage from its structure and content, which is defined using HTML (Hypertext Markup Language).
Benefits of Using Via Style
Using via style offers several benefits in web development:
-
Consistency: Via style ensures a consistent visual appearance across different pages or sections of a website. By defining styles in a central CSS file, you can maintain a cohesive look and feel throughout your application.
-
Reusability: Styles defined using CSS can be reused across multiple elements or pages. This promotes code reuse and reduces duplication, making your codebase more maintainable.
-
Separation of Concerns: Via style allows you to separate the presentation layer from the structure and functionality of your application. This separation makes it easier to update and modify styles without affecting the underlying code.
-
Flexibility: CSS provides a wide range of styling options, allowing you to customize the appearance of your application to match your desired design. You can easily change colors, fonts, layouts, and other visual aspects without modifying the HTML structure.
Interactive Route and its Limitations
An interactive route, also known as a dynamic route or a route with parameters, is a URL pattern that contains dynamic segments. These segments can vary based on user input or data from a database, allowing for more flexible and personalized URLs.
Here’s an example of an interactive route:
/products/:id
In this example, :id
is a dynamic segment that can be replaced with an actual value, such as /products/123
or /products/abc
.
While interactive routes provide powerful capabilities for creating dynamic web applications, they can impose certain limitations when it comes to styling.
Limitations of Via Style with Interactive Routes
-
Dynamic Class Names: When using interactive routes, the class names of elements may be generated dynamically based on the route parameters. This can make it challenging to target specific elements using CSS selectors, as the class names may change depending on the route.
-
Scoped Styles: Interactive routes often involve rendering components or views based on the route parameters. These components may have their own scoped styles, which can override or conflict with the global via styles defined in your CSS file.
-
Server-Side Rendering: If your application uses server-side rendering (SSR) with interactive routes, the initial HTML sent from the server may not include the dynamically generated class names. This can lead to a mismatch between the server-rendered HTML and the client-side styles, resulting in a flash of unstyled content (FOUC).
-
Style Encapsulation: Some web development frameworks or libraries, such as Vue.js or Angular, provide component-based architectures with built-in style encapsulation. In these cases, the styles defined within a component may not be easily overridden by global via styles.
Techniques for Managing Via Style with Interactive Routes
Despite the limitations, there are techniques you can employ to manage via style effectively when using interactive routes:
- CSS Modules: CSS Modules allow you to write modular and scoped CSS styles that are specific to a component. By importing and using these modules in your components, you can ensure that styles are encapsulated and won’t conflict with other parts of your application.
Example of using CSS Modules:
“`jsx
import styles from ‘./MyComponent.module.css’;
function MyComponent() {
return
;
}
“`
- Inline Styles: Instead of relying on external CSS files, you can use inline styles to apply styles directly to elements. Inline styles are defined using the
style
attribute in HTML or the equivalent property in JavaScript frameworks.
Example of using inline styles:
“`jsx
function MyComponent() {
const myStyle = {
color: ‘red’,
fontSize: ’16px’,
};
return <div style={myStyle}>Hello, world!</div>;
}
“`
- CSS-in-JS Libraries: There are various CSS-in-JS libraries available, such as styled-components or emotion, that allow you to write CSS directly in your JavaScript code. These libraries provide a way to define styles that are scoped to specific components and can be easily integrated with interactive routes.
Example of using styled-components:
“`jsx
import styled from ‘styled-components’;
const MyStyledComponent = styled.divcolor: red;
;
font-size: 16px;
function MyComponent() {
return
}
“`
- Global CSS with Specific Selectors: If you prefer to use global CSS files, you can still target specific elements within interactive routes by using more specific CSS selectors. This can involve using a combination of class names, data attributes, or hierarchical selectors to precisely target the desired elements.
Example of using specific selectors:
css
.product-page .product-title {
font-size: 24px;
color: blue;
}
jsx
function ProductPage({ productId }) {
return (
<div className="product-page">
<h2 className="product-title">Product {productId}</h2>
{/* ... */}
</div>
);
}
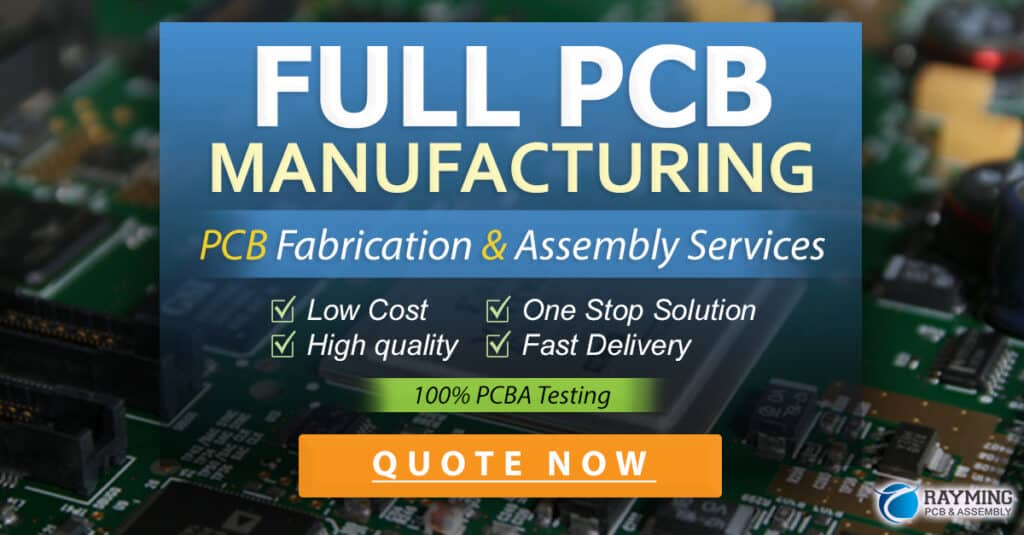
Strategies for Consistent Via Style
To maintain a consistent via style across your application, even with interactive routes, consider the following strategies:
-
Define a Style Guide: Create a style guide that outlines the overall visual design and branding of your application. This guide should include color palettes, typography, spacing, and other design elements that should be consistently applied throughout your application.
-
Use CSS Variables: CSS variables, also known as CSS custom properties, allow you to define reusable values that can be referenced throughout your stylesheets. By defining variables for colors, fonts, and other commonly used values, you can ensure consistency and make it easier to update styles globally.
Example of using CSS variables:
“`css
:root {
–primary-color: #007bff;
–font-size-base: 16px;
}
.button {
background-color: var(–primary-color);
font-size: var(–font-size-base);
}
“`
-
Implement a Design System: A design system is a collection of reusable components, patterns, and guidelines that define the visual language and interaction patterns of your application. By building a design system, you can ensure consistency across different routes and components, as well as streamline the development process.
-
Use Pre-processors or Post-processors: CSS pre-processors like Sass or Less, or post-processors like PostCSS, offer additional features and capabilities that can help manage and organize your styles. These tools allow you to use variables, mixins, nesting, and other techniques to write more modular and maintainable CSS.
-
Regularly Review and Refactor: Periodically review your codebase and refactor your styles to ensure consistency and adherence to your style guide. Remove any unused or redundant styles, and consolidate similar styles to maintain a clean and organized stylesheet.
FAQ
-
What is via style?
Via style refers to the visual styling and appearance of elements in a user interface or webpage, controlled using CSS. -
What are the benefits of using via style?
The benefits of using via style include consistency, reusability, separation of concerns, and flexibility in designing the visual appearance of a web application. -
What is an interactive route?
An interactive route, also known as a dynamic route or a route with parameters, is a URL pattern that contains dynamic segments that can vary based on user input or data from a database. -
What are the limitations of via style with interactive routes?
The limitations of via style with interactive routes include dynamic class names, scoped styles, server-side rendering challenges, and style encapsulation in component-based architectures. -
What are some techniques for managing via style with interactive routes?
Techniques for managing via style with interactive routes include using CSS Modules, inline styles, CSS-in-JS libraries, and targeting specific elements with more precise CSS selectors.
Conclusion
Managing via style while using interactive routes can present challenges, but with the right techniques and strategies, you can maintain a consistent and visually appealing user interface. By leveraging CSS Modules, inline styles, CSS-in-JS libraries, or specific selectors, you can overcome the limitations posed by dynamic routes.
Additionally, defining a style guide, using CSS variables, implementing a design system, and regularly reviewing and refactoring your styles can help ensure consistency and maintainability across your application.
Remember, the key is to find a balance between the flexibility of interactive routes and the maintainability and consistency of your via styles. By employing best practices and choosing the appropriate techniques for your project, you can create a seamless and visually cohesive user experience.
Technique | Description |
---|---|
CSS Modules | Write modular and scoped CSS styles specific to a component |
Inline Styles | Apply styles directly to elements using the style attribute or equivalent property |
CSS-in-JS Libraries | Write CSS directly in JavaScript code using libraries like styled-components or emotion |
Global CSS with Selectors | Use specific CSS selectors to target elements within interactive routes |
CSS Variables | Define reusable values that can be referenced throughout stylesheets for consistency |
Design System | Build a collection of reusable components, patterns, and guidelines for a consistent visual language |
By understanding the limitations and employing the appropriate techniques, you can successfully manage via style while leveraging the power of interactive routes in your web application.
Leave a Reply