Introduction to Brushless Motor Controllers
Brushless motor controllers are essential components in various applications, from robotics and drones to electric vehicles and industrial automation. These controllers regulate the speed and torque of brushless DC (BLDC) motors by precisely controlling the power delivered to the motor windings. In this article, we will delve into the process of building an advanced brushless motor controller, covering the fundamental concepts, hardware components, software implementation, and practical considerations.
Understanding Brushless DC Motors
Before we start building a brushless motor controller, it’s crucial to understand how BLDC motors work. Unlike traditional brushed DC motors, BLDC motors do not have physical brushes and commutators. Instead, they rely on electronic commutation, where the controller determines the timing and sequence of energizing the motor windings to create a rotating magnetic field.
BLDC motors consist of two main parts:
1. Stator: The stationary part of the motor, which contains the windings.
2. Rotor: The rotating part of the motor, which contains permanent magnets.
The number of poles in the rotor and the number of phases in the stator determine the motor’s characteristics and performance.
Advantages of Brushless Motors
Brushless motors offer several advantages over their brushed counterparts:
– Higher efficiency due to the absence of brushes and commutator
– Longer lifespan as there are no brushes to wear out
– Lower maintenance requirements
– Higher power density and better heat dissipation
– Smoother operation and less electromagnetic interference (EMI)
These advantages make brushless motors an attractive choice for many applications, particularly those requiring high performance and reliability.
Brushless Motor Controller Components
To build an advanced brushless motor controller, you’ll need the following key components:
Microcontroller or DSP
The heart of the brushless motor controller is a microcontroller or digital signal processor (DSP). This device executes the control algorithms, generates the necessary PWM signals, and interfaces with sensors and communication modules. Popular choices include:
– Arduino boards (e.g., Arduino Uno, Arduino Mega)
– STM32 microcontrollers
– Texas Instruments C2000 series DSPs
When selecting a microcontroller or DSP, consider factors such as processing power, memory, peripheral interfaces, and ease of programming.
Motor Driver
The motor driver is responsible for amplifying the low-power control signals from the microcontroller and driving the high-power motor windings. It typically consists of a three-phase inverter bridge made up of power MOSFETs or IGBTs. The motor driver also includes gate drivers to ensure proper switching of the power devices.
Some commonly used motor driver ICs are:
– IR2110 high and low side driver
– DRV8301 three-phase gate driver
– L6234 three-phase motor driver
Choose a motor driver based on the voltage and current requirements of your BLDC motor, as well as the desired features like overcurrent protection and temperature monitoring.
Current Sensing
Current sensing is essential for closed-loop control of the brushless motor. By measuring the current flowing through the motor windings, the controller can implement torque control, overcurrent protection, and other advanced features. There are several methods for current sensing:
– Shunt resistors: Low-value resistors placed in series with the motor windings, with the voltage drop measured using differential amplifiers.
– Hall-effect current sensors: Non-intrusive sensors that measure the magnetic field generated by the current flowing through a conductor.
– Integrated current sensing: Some motor driver ICs have built-in current sensing capabilities.
Consider the accuracy, bandwidth, and isolation requirements when selecting a current sensing method.
Position Sensing
To achieve precise control of the brushless motor, the controller needs to know the rotor’s position at any given time. There are two common methods for position sensing:
1. Hall-effect sensors: These sensors detect the magnetic field of the rotor’s permanent magnets and provide discrete position information (e.g., 60° intervals for a three-phase motor).
2. Encoders: Optical or magnetic encoders provide high-resolution position feedback, enabling more accurate control and smoother operation.
Choose a position sensing method based on the required resolution, cost, and compatibility with your motor and mechanical setup.
Power Supply
The brushless motor controller requires a stable and regulated power supply to operate reliably. The power supply should match the voltage and current requirements of your motor and controller components. Consider using a switching regulator (e.g., buck converter) to efficiently step down the voltage from a higher voltage source, such as a battery pack.
Include appropriate filtering capacitors and voltage protection circuits to ensure a clean and robust power supply.
Communication Interfaces
To interface with higher-level control systems or user interfaces, the brushless motor controller may include communication modules such as:
– UART (Universal Asynchronous Receiver/Transmitter)
– I2C (Inter-Integrated Circuit)
– SPI (Serial Peripheral Interface)
– CAN (Controller Area Network)
Choose a communication interface that is compatible with your overall system architecture and meets the bandwidth and reliability requirements.
Brushless Motor Control Techniques
There are various control techniques for brushless motors, each with its own advantages and complexities. Let’s explore some of the most common techniques:
Trapezoidal Control (Six-Step Commutation)
Trapezoidal control, also known as six-step commutation, is a simple and widely used technique for brushless motor control. It involves energizing the motor windings in a specific sequence based on the rotor position, creating a trapezoidal back-EMF waveform.
The six-step commutation sequence is as follows:
Step | Phase A | Phase B | Phase C |
---|---|---|---|
1 | + | – | OFF |
2 | + | OFF | – |
3 | OFF | + | – |
4 | – | + | OFF |
5 | – | OFF | + |
6 | OFF | – | + |
To implement trapezoidal control, the controller uses the position feedback from Hall-effect sensors to determine the appropriate commutation step. The PWM signals are then generated to drive the motor windings accordingly.
Trapezoidal control is relatively simple to implement and offers good performance for many applications. However, it can suffer from torque ripple and audible noise due to the discrete nature of the commutation steps.
Sinusoidal Control (Field-Oriented Control)
Sinusoidal control, also known as field-oriented control (FOC), is a more advanced technique that aims to achieve smoother operation and reduced torque ripple compared to trapezoidal control. In sinusoidal control, the controller generates sinusoidal current waveforms that are synchronized with the rotor’s magnetic field.
The key concepts in sinusoidal control are:
– Clarke transform: Converts the three-phase stator currents (ia, ib, ic) into a two-phase stationary reference frame (iα, iβ).
– Park transform: Converts the two-phase stationary reference frame (iα, iβ) into a rotating reference frame (id, iq) aligned with the rotor’s magnetic field.
– Inverse Park transform: Converts the rotating reference frame (id, iq) back to the stationary reference frame (iα, iβ).
– Space vector modulation (SVM): Generates the appropriate PWM signals based on the desired voltage vectors in the stationary reference frame.
Sinusoidal control requires accurate position feedback, typically obtained from encoders or high-resolution magnetic sensors. The control algorithm involves complex mathematical transformations and PI (Proportional-Integral) controllers to regulate the current in the rotating reference frame.
While sinusoidal control is more computationally intensive than trapezoidal control, it offers several advantages:
– Smoother torque output and reduced torque ripple
– Lower acoustic noise and vibration
– Better efficiency and dynamic performance
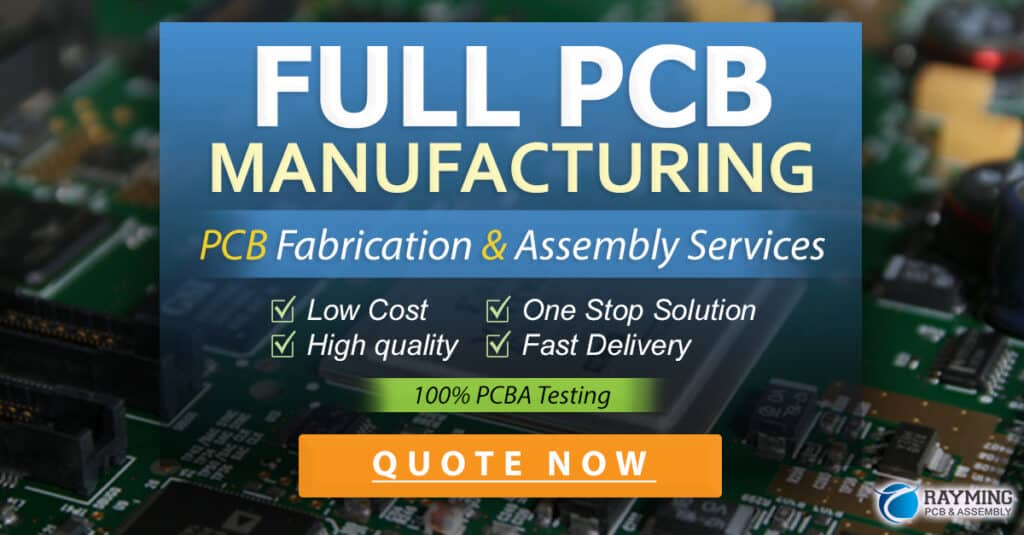
Software Implementation
To implement the brushless motor control techniques discussed above, you’ll need to develop software for your microcontroller or DSP. The software should perform the following tasks:
1. Initialize and configure the hardware peripherals (e.g., PWM, ADC, communication interfaces).
2. Read the position feedback from sensors (Hall-effect sensors or encoders).
3. Execute the control algorithm (trapezoidal or sinusoidal) based on the position feedback and desired speed or torque setpoint.
4. Generate the appropriate PWM signals to drive the motor windings.
5. Implement safety features such as overcurrent protection, overvoltage protection, and fault handling.
6. Communicate with external systems or user interfaces.
Here’s a simplified example of trapezoidal control using an Arduino board:
// Define motor pins
const int MOTOR_PIN_A = 9;
const int MOTOR_PIN_B = 10;
const int MOTOR_PIN_C = 11;
// Define Hall-effect sensor pins
const int HALL_A_PIN = 2;
const int HALL_B_PIN = 3;
const int HALL_C_PIN = 4;
// Variables for commutation sequence
int hallState = 0;
int commutationStep = 0;
void setup() {
// Initialize motor pins as outputs
pinMode(MOTOR_PIN_A, OUTPUT);
pinMode(MOTOR_PIN_B, OUTPUT);
pinMode(MOTOR_PIN_C, OUTPUT);
// Initialize Hall-effect sensor pins as inputs
pinMode(HALL_A_PIN, INPUT);
pinMode(HALL_B_PIN, INPUT);
pinMode(HALL_C_PIN, INPUT);
}
void loop() {
// Read Hall-effect sensor states
int hallA = digitalRead(HALL_A_PIN);
int hallB = digitalRead(HALL_B_PIN);
int hallC = digitalRead(HALL_C_PIN);
// Determine commutation step based on Hall-effect sensor states
hallState = (hallA << 2) | (hallB << 1) | hallC;
switch (hallState) {
case 1:
commutationStep = 0;
break;
case 3:
commutationStep = 1;
break;
case 2:
commutationStep = 2;
break;
case 6:
commutationStep = 3;
break;
case 4:
commutationStep = 4;
break;
case 5:
commutationStep = 5;
break;
}
// Perform commutation based on the current step
switch (commutationStep) {
case 0:
digitalWrite(MOTOR_PIN_A, HIGH);
digitalWrite(MOTOR_PIN_B, LOW);
digitalWrite(MOTOR_PIN_C, LOW);
break;
case 1:
digitalWrite(MOTOR_PIN_A, HIGH);
digitalWrite(MOTOR_PIN_B, LOW);
digitalWrite(MOTOR_PIN_C, HIGH);
break;
case 2:
digitalWrite(MOTOR_PIN_A, LOW);
digitalWrite(MOTOR_PIN_B, LOW);
digitalWrite(MOTOR_PIN_C, HIGH);
break;
case 3:
digitalWrite(MOTOR_PIN_A, LOW);
digitalWrite(MOTOR_PIN_B, HIGH);
digitalWrite(MOTOR_PIN_C, HIGH);
break;
case 4:
digitalWrite(MOTOR_PIN_A, LOW);
digitalWrite(MOTOR_PIN_B, HIGH);
digitalWrite(MOTOR_PIN_C, LOW);
break;
case 5:
digitalWrite(MOTOR_PIN_A, HIGH);
digitalWrite(MOTOR_PIN_B, HIGH);
digitalWrite(MOTOR_PIN_C, LOW);
break;
}
}
This example demonstrates a basic implementation of trapezoidal control using Hall-effect sensors. The code reads the sensor states, determines the appropriate commutation step, and energizes the motor windings accordingly.
For more advanced control techniques like sinusoidal control, you’ll need to use a more powerful microcontroller or DSP and implement the necessary mathematical transformations and control algorithms.
Practical Considerations
When building an advanced brushless motor controller, there are several practical considerations to keep in mind:
PCB Design
Designing a robust and reliable printed circuit board (PCB) is crucial for the controller’s performance and longevity. Consider the following factors:
– Proper layout and grounding to minimize electromagnetic interference (EMI) and noise.
– Adequate trace widths and copper thickness to handle the expected currents.
– Proper heat dissipation for power components like MOSFETs and voltage regulators.
– Appropriate connectors and terminals for power input, motor output, and sensor interfaces.
Enclosure and Cooling
The brushless motor controller should be housed in a suitable enclosure to protect the electronics from dust, moisture, and mechanical damage. Consider the operating environment and choose an enclosure with the appropriate ingress protection (IP) rating.
If the controller generates significant heat, incorporate cooling solutions such as heatsinks, fans, or liquid cooling to ensure reliable operation and prevent thermal shutdown.
Firmware Development and Debugging
Developing and debugging the firmware for the brushless motor controller can be challenging, especially when implementing complex control algorithms. Use a development environment that provides debugging tools and supports your chosen microcontroller or DSP.
Consider using real-time operating systems (RTOS) or middleware libraries to simplify task scheduling, inter-process communication, and peripheral management.
Testing and Validation
Before deploying the brushless motor controller in a real-world application, thoroughly test and validate its performance and safety features. Conduct tests under various operating conditions, including:
– Different load scenarios (no-load, rated load, overload)
– Temperature variations
– Power supply fluctuations
– Fault conditions (e.g., overcurrent, overvoltage, sensor failure)
Use appropriate test equipment such as oscilloscopes, current probes, and data loggers to monitor and analyze the controller’s behavior.
Frequently Asked Questions (FAQ)
Q1: Can I use a brushless motor controller with any BLDC motor?
A1: In general, brushless motor controllers are designed to work with a wide range of BLDC motors. However, it’s essential to ensure that the controller’s specifications (voltage range, current rating, etc.) match the requirements of your specific motor. Additionally, the controller should be compatible with the motor’s position sensing method (Hall-effect sensors or encoders).
Q2: What is the difference between sensorless and sensored brushless motor control?
A2: Sensorless brushless motor control eliminates the need for position sensors (Hall-effect sensors or encoders) by estimating the rotor position based on the back-EMF voltage induced in the motor windings. This approach reduces the cost and complexity of the system but may have limitations in low-speed operation and startup. Sensored control, on the other hand, relies on dedicated position sensors for accurate rotor position feedback, enabling precise control throughout the entire speed range.
Q3: How do I tune the PID parameters for optimal motor performance?
A3: Tuning the PID (Proportional-Integral-Derivative) parameters for optimal motor performance involves finding the right balance between responsiveness, stability, and accuracy. Start by setting the integral and derivative gains to zero and gradually increase the proportional gain until the motor responds quickly without oscillations. Then, introduce a small integral gain to eliminate steady-state error and improve low-speed performance. Finally, add a small derivative gain to enhance stability and damping. Fine-tune the parameters through iterative testing and observation.
Q4: What safety features should I include in my brushless motor controller?
A4: To ensure safe and reliable operation, include the following safety features in your brushless motor controller:
– Overcurrent protection: Monitor the motor current and shut down the controller if it exceeds a predefined threshold.
– Overvoltage protection: Protect the controller and motor from voltage spikes using transient voltage suppressors (TVS) or varistors.
– Undervoltage lockout: Prevent the controller from operating if the power supply voltage drops below a safe level.
– Thermal protection: Monitor the temperature of critical components (e.g., MOSFETs, microcontroller) and implement thermal shutdown if necessary.
– Failsafe modes: Define safe operating modes in case of sensor failures, communication errors, or other faults.
Q5: Can I control multiple brushless motors with a single controller?
A5: Yes, it is possible to control multiple brushless motors with a single controller, depending on the controller’s capabilities and the system requirements. Some advanced controllers feature multiple motor channels, allowing independent control of several motors. However, controlling multiple motors requires careful design considerations, such as ensuring sufficient processing power, memory, and I/O resources. Additionally, you’ll need to implement appropriate synchronization and coordination mechanisms to achieve the desired multi-motor behavior.
Conclusion
Building an advanced brushless motor controller involves a combination of hardware design, software development, and control theory. By understanding the fundamental concepts, selecting the right components, and implementing appropriate control techniques, you can create a high-performance controller tailored to your specific application requirements.
Remember to consider practical aspects such as PCB design, enclosure, cooling, and testing to ensure the controller’s reliability and longevity. Continuously iterate and improve your design based on real-world feedback and performance data.
As you delve deeper into the world of brushless motor control, keep exploring new technologies, algorithms, and best practices to
Leave a Reply