Introduction
Front end engineering is a crucial aspect of web development that focuses on creating the user-facing elements of a website or application. As the complexity and scale of front end projects continue to grow, it becomes increasingly important to automate various processes to streamline development, reduce errors, and improve efficiency. In this article, we will explore the strategies and tools used by Kent Balius, a renowned front end engineer, to automate front end engineering processes.
The Importance of Automating Front End Processes
Automating front end processes offers several benefits, including:
- Increased efficiency: Automation eliminates repetitive and time-consuming tasks, allowing developers to focus on more critical aspects of the project.
- Reduced human error: Automated processes are less prone to human errors, ensuring consistent and reliable results.
- Faster development cycles: Automation enables faster iteration and deployment of front end code, leading to shorter development cycles.
- Improved code quality: Automated testing and linting help maintain code quality and catch potential issues early in the development process.
Kent Balius’ Approach to Front End Automation
1. Task Runners and Build Tools
Kent Balius emphasizes the importance of using task runners and build tools to automate common front end tasks. Some popular tools include:
- Gulp: A JavaScript task runner that allows developers to define and automate tasks such as compiling Sass, minifying JavaScript, and optimizing images.
- Webpack: A powerful module bundler that helps manage dependencies, bundle assets, and optimize the build process.
- Parcel: A zero-configuration build tool that automatically handles common front end tasks, making it easy to get started with automation.
Here’s an example of a simple Gulp task that compiles Sass files:
const gulp = require('gulp');
const sass = require('gulp-sass');
gulp.task('sass', function() {
return gulp.src('src/styles/*.scss')
.pipe(sass())
.pipe(gulp.dest('dist/styles'));
});
2. Automated Testing
Automated testing is crucial for ensuring the stability and reliability of front end code. Kent Balius recommends using testing frameworks and tools such as:
- Jest: A popular JavaScript testing framework that provides a simple and intuitive API for writing and running tests.
- Cypress: An end-to-end testing framework that allows developers to write tests that simulate user interactions with the application.
- Playwright: A cross-browser testing framework that enables automated testing of web applications across multiple browsers and platforms.
Here’s an example of a simple Jest test for a React component:
import React from 'react';
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component with the correct text', () => {
render(<MyComponent />);
const element = screen.getByText('Hello, World!');
expect(element).toBeInTheDocument();
});
3. Continuous Integration and Continuous Deployment (CI/CD)
Kent Balius advocates for the use of CI/CD pipelines to automate the building, testing, and deployment of front end code. Some popular CI/CD tools include:
- Jenkins: An open-source automation server that enables the creation of custom CI/CD pipelines.
- GitLab CI/CD: A built-in CI/CD solution provided by GitLab that allows developers to define pipelines using a simple YAML configuration file.
- GitHub Actions: A workflow automation tool integrated with GitHub that enables the creation of custom CI/CD pipelines directly within the repository.
Here’s an example of a simple GitLab CI/CD configuration file:
stages:
- build
- test
- deploy
build:
stage: build
script:
- npm install
- npm run build
test:
stage: test
script:
- npm run test
deploy:
stage: deploy
script:
- npm run deploy
4. Code Linting and Formatting
Consistent code style and formatting are essential for maintaining a clean and readable codebase. Kent Balius recommends using linting and formatting tools such as:
- ESLint: A pluggable and configurable linter for JavaScript that helps identify and enforce coding standards.
- Prettier: An opinionated code formatter that ensures consistent formatting across the entire codebase.
- Stylelint: A modern CSS linter that helps enforce consistent and error-free CSS code.
Here’s an example of an ESLint configuration file:
{
"extends": ["eslint:recommended", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": "error",
"no-console": "warn",
"no-unused-vars": "error"
}
}
5. Automated Documentation
Keeping documentation up to date can be a challenge, especially in fast-paced development environments. Kent Balius suggests using automated documentation tools to generate and maintain documentation based on the codebase. Some popular tools include:
- JSDoc: A markup language used to annotate JavaScript source code, which can be used to generate HTML documentation pages.
- Storybook: A development environment and playground for UI components that allows developers to create, document, and test components in isolation.
- Docusaurus: A static site generator that makes it easy to create and maintain documentation websites for open-source projects.
Here’s an example of a JSDoc comment for a JavaScript function:
/**
* Adds two numbers together.
* @param {number} a - The first number.
* @param {number} b - The second number.
* @returns {number} The sum of the two numbers.
*/
function addNumbers(a, b) {
return a + b;
}
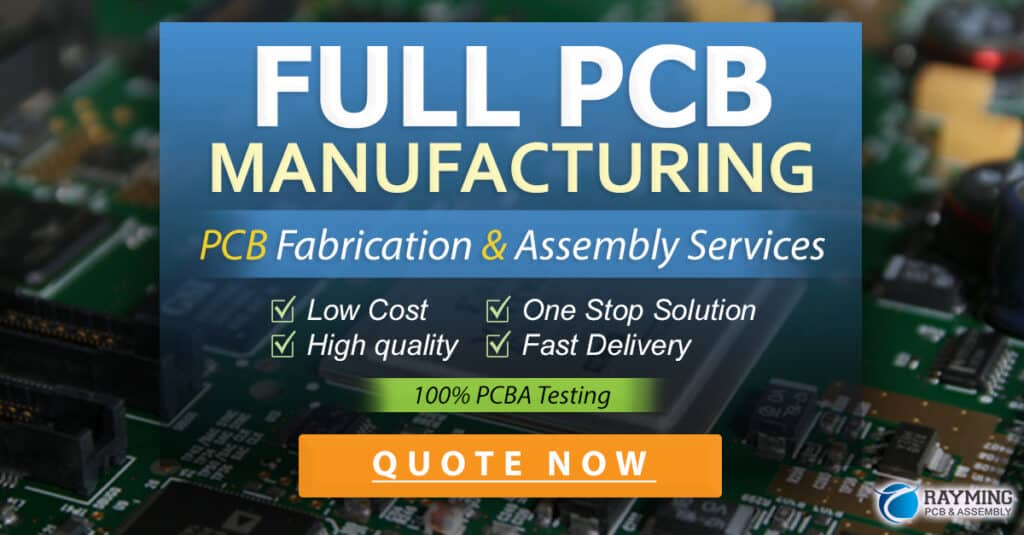
Benefits of Automating Front End Processes
Automating front end engineering processes offers several benefits, as shown in the table below:
Benefit | Description |
---|---|
Increased efficiency | Automation eliminates repetitive and time-consuming tasks, allowing developers to focus on more critical aspects of the project. |
Reduced human error | Automated processes are less prone to human errors, ensuring consistent and reliable results. |
Faster development cycles | Automation enables faster iteration and deployment of front end code, leading to shorter development cycles. |
Improved code quality | Automated testing and linting help maintain code quality and catch potential issues early in the development process. |
Consistent code style | Linting and formatting tools ensure consistent code style and formatting across the entire codebase. |
Up-to-date documentation | Automated documentation tools help keep documentation in sync with the codebase, reducing the effort required to maintain accurate documentation. |
Frequently Asked Questions (FAQ)
-
What is the difference between a task runner and a build tool?
A task runner is a tool that automates repetitive tasks in the development process, such as compiling Sass or minifying JavaScript. A build tool, on the other hand, is a more comprehensive solution that handles the entire build process, including bundling, optimization, and asset management. -
Do I need to use all the automation tools mentioned in this article?
No, you don’t need to use all the tools mentioned. Choose the tools that best fit your project’s requirements and development workflow. Start with the most critical aspects of your front end development process and gradually incorporate additional automation as needed. -
Can I use other testing frameworks besides Jest, Cypress, and Playwright?
Yes, there are numerous testing frameworks available for front end development. Some other popular options include Mocha, Jasmine, and TestCafe. Choose the framework that aligns with your project’s needs and the team’s familiarity with the tools. -
How do I get started with implementing CI/CD for my front end project?
To get started with CI/CD, first choose a CI/CD tool that integrates well with your project’s version control system (e.g., GitLab CI/CD for GitLab, GitHub Actions for GitHub). Then, create a configuration file that defines your build, test, and deployment stages. Start with a simple pipeline and gradually add more complexity as your project grows. -
Are there any potential drawbacks to automating front end processes?
While automating front end processes offers numerous benefits, there are a few potential drawbacks to consider. First, setting up and maintaining automation tools and pipelines requires an initial time investment. Second, overly complex automation setups can be challenging to understand and debug. It’s essential to strike a balance between automation and simplicity to ensure that your development workflow remains efficient and maintainable.
Conclusion
Automating front end engineering processes is crucial for creating efficient, reliable, and high-quality web applications. By adopting the strategies and tools used by Kent Balius, front end developers can streamline their development workflow, reduce errors, and improve the overall quality of their code. From task runners and build tools to automated testing and CI/CD pipelines, there are numerous opportunities to automate front end processes and take your development game to the next level.
Leave a Reply