Understanding the Problem of Copying Multiline Text
When working with text editors, IDEs, or any application that involves copying and pasting text, you may have encountered a frustrating issue: copying multiline text and trying to paste it into a string variable only results in the first line being pasted. This problem can be confusing and time-consuming, especially if you’re dealing with large amounts of text or need to preserve the original formatting.
In this article, we will explore the reasons behind this behavior and provide solutions to ensure that you can successfully copy and paste multiline text into string variables without losing any information.
What Causes the First Line Only to be Pasted?
To understand why only the first line gets pasted when copying multiline text, let’s dive into how different programming languages and environments handle strings and line breaks.
String Literal Syntax
In many programming languages, string literals are defined using either single quotes ('
) or double quotes ("
). For example:
my_string = "Hello, world!"
However, when you have a string that spans multiple lines, you need to use a different syntax to preserve the line breaks.
Line Breaks in Strings
Line breaks in strings are represented differently depending on the operating system:
– Windows uses the sequence \r\n
(carriage return followed by a newline)
– Unix-based systems (Linux and macOS) use \n
(newline)
– Classic Mac OS (pre-OS X) used \r
(carriage return)
When you copy multiline text and paste it into a string literal, the line breaks are usually not automatically converted to the appropriate syntax. As a result, the string literal only includes the first line of the copied text.
Solutions for Copying Multiline Text
Now that we understand the problem, let’s explore various solutions to ensure that multiline text is correctly pasted into string variables.
Solution 1: Using Triple Quotes
Many programming languages support triple quotes ('''
or """
) for defining multiline strings. Triple quotes allow you to include line breaks directly in the string literal without any additional syntax. Here’s an example in Python:
multiline_string = '''
This is a
multiline string
using triple quotes.
'''
By using triple quotes, you can easily paste multiline text into the string literal, and the line breaks will be preserved.
Solution 2: Concatenating Lines with Line Break Characters
Another approach is to concatenate the lines of the multiline text using the appropriate line break character for your operating system. Here’s an example in JavaScript:
const multilineString = "This is line 1\n" +
"This is line 2\n" +
"This is line 3";
In this example, we use the \n
character to represent line breaks and concatenate the lines using the +
operator. This method allows you to manually format the multiline string while ensuring that the line breaks are preserved.
Solution 3: Using String Interpolation or Template Literals
Some programming languages provide string interpolation or template literals, which allow you to include expressions and line breaks within a string. Here’s an example in Python using f-strings (formatted string literals):
line1 = "This is line 1"
line2 = "This is line 2"
line3 = "This is line 3"
multiline_string = f"""
{line1}
{line2}
{line3}
"""
In this example, we use f-strings to define a multiline string and include variables within the string using curly braces {}
. The line breaks are preserved, and the variables are interpolated into the resulting string.
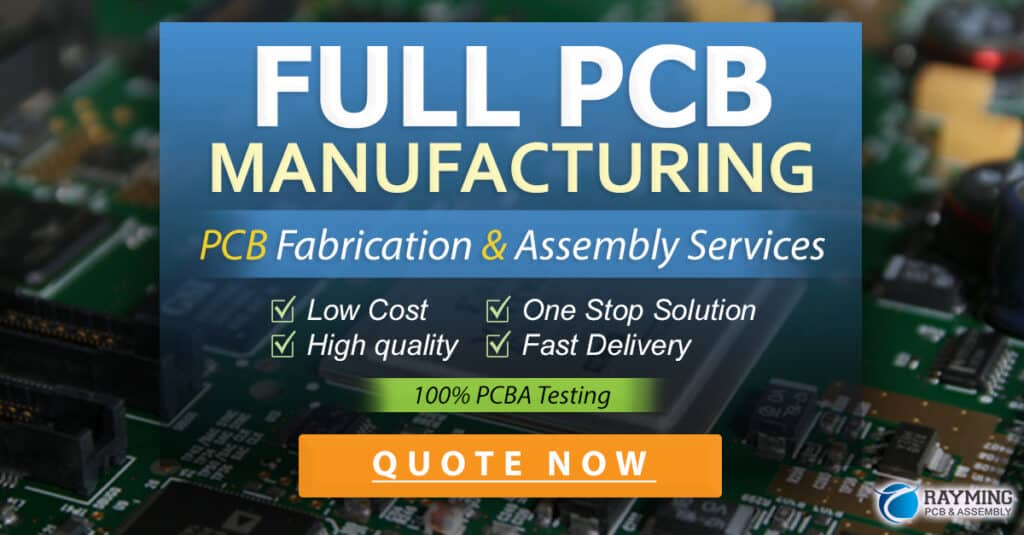
Best Practices for Handling Multiline Text
To ensure a smooth experience when working with multiline text, consider the following best practices:
-
Choose the appropriate solution: Depending on your programming language and requirements, select the solution that best fits your needs. Triple quotes, concatenation with line break characters, or string interpolation are all viable options.
-
Be consistent with line break characters: When manually concatenating lines or using line break characters, make sure to use the appropriate character for your operating system (
\n
for Unix-based systems,\r\n
for Windows) to ensure compatibility. -
Use text editors with multi-line string support: Some text editors and IDEs provide built-in support for multi-line strings, making it easier to paste and format multiline text. Look for features like “Paste as String” or “Paste as Multiline String” in your editor’s options.
-
Escape special characters if necessary: If your multiline text contains special characters like quotes or backslashes, make sure to escape them properly to avoid syntax errors. Use backslashes (
\
) to escape characters within the string. -
Test and validate the resulting string: After pasting multiline text into a string variable, always test and validate the resulting string to ensure that it matches your expected format and content. Print the string or use debugging tools to verify that the line breaks and content are preserved correctly.
Copying Multiline Text in Different Programming Languages
Now let’s explore how to handle copying multiline text in some popular programming languages.
Python
In Python, you can use triple quotes ('''
or """
) to define multiline strings:
multiline_string = '''
This is a
multiline string
in Python.
'''
Alternatively, you can use concatenation with the \n
character:
multiline_string = "This is line 1\n" \
"This is line 2\n" \
"This is line 3"
Python also supports f-strings for string interpolation:
line1 = "This is line 1"
line2 = "This is line 2"
line3 = "This is line 3"
multiline_string = f"""
{line1}
{line2}
{line3}
"""
JavaScript
In JavaScript, you can use the +
operator to concatenate lines with the \n
character:
const multilineString = "This is line 1\n" +
"This is line 2\n" +
"This is line 3";
JavaScript also supports template literals (backticks) for string interpolation:
const line1 = "This is line 1";
const line2 = "This is line 2";
const line3 = "This is line 3";
const multilineString = `
${line1}
${line2}
${line3}
`;
Java
In Java, you can use the +
operator to concatenate lines with the \n
character:
String multilineString = "This is line 1\n" +
"This is line 2\n" +
"This is line 3";
Java also supports text blocks (triple double quotes) starting from Java 15:
String multilineString = """
This is line 1
This is line 2
This is line 3
""";
C
In C#, you can use the @
symbol before a string literal to define a verbatim string, which allows you to include line breaks:
string multilineString = @"
This is line 1
This is line 2
This is line 3
";
You can also use concatenation with the \n
character:
string multilineString = "This is line 1\n" +
"This is line 2\n" +
"This is line 3";
Language | Solution |
---|---|
Python | Triple quotes, concatenation with \n , f-strings |
JavaScript | Concatenation with \n , template literals |
Java | Concatenation with \n , text blocks (Java 15+) |
C# | Verbatim strings with @ , concatenation with \n |
FAQ
1. Why does copying multiline text and pasting it into a string only include the first line?
When you copy multiline text and paste it into a string literal, the line breaks are usually not automatically converted to the appropriate syntax for the programming language. As a result, the string literal only includes the first line of the copied text.
2. What are the different ways to handle multiline text in strings?
There are several ways to handle multiline text in strings:
– Using triple quotes ('''
or """
) to define multiline strings directly.
– Concatenating lines with the appropriate line break character (\n
for Unix-based systems, \r\n
for Windows).
– Using string interpolation or template literals to include expressions and line breaks within a string.
3. How can I ensure that the line breaks are preserved when pasting multiline text?
To ensure that the line breaks are preserved when pasting multiline text, you can use one of the following methods:
– Use triple quotes to define the multiline string, allowing you to include line breaks directly.
– Manually concatenate the lines using the appropriate line break character for your operating system.
– Use string interpolation or template literals to include line breaks within the string.
4. Are there any best practices for handling multiline text in strings?
Yes, some best practices for handling multiline text in strings include:
– Choosing the appropriate solution based on your programming language and requirements.
– Being consistent with line break characters and using the appropriate character for your operating system.
– Using text editors or IDEs with built-in support for multi-line strings.
– Escaping special characters if necessary to avoid syntax errors.
– Testing and validating the resulting string to ensure that the line breaks and content are preserved correctly.
5. Can I copy and paste multiline text into strings in any programming language?
Most programming languages provide ways to handle multiline text in strings. Some common approaches include using triple quotes, concatenating lines with line break characters, or using string interpolation or template literals. However, the specific syntax and features may vary depending on the programming language you are using. It’s important to refer to the documentation or examples specific to your language to determine the best approach.
Conclusion
Copying multiline text and pasting it into a string variable can be frustrating when only the first line gets included. However, by understanding the reasons behind this behavior and utilizing the appropriate solutions, you can ensure that multiline text is correctly pasted and preserved in your strings.
Whether you choose to use triple quotes, concatenate lines with line break characters, or leverage string interpolation or template literals, the key is to be consistent and follow best practices to maintain readability and compatibility.
Remember to test and validate your strings after pasting multiline text to ensure that the content and formatting are as expected. By doing so, you can confidently work with multiline text in your programs and avoid any surprises or data loss.
With the knowledge gained from this article, you are now equipped to handle multiline text copying and pasting efficiently and effectively in your programming projects.
Leave a Reply