Introduction to Arduino Custom Libraries
Arduino is an open-source electronics platform that has revolutionized the world of hobbyist electronics and prototyping. One of the key features of Arduino is its extensibility through the use of custom libraries. These libraries allow users to extend the functionality of the Arduino platform by providing additional functions and features that are not included in the core Arduino software.
In this article, we will explore the concept of Arduino custom libraries in detail. We will discuss what they are, why they are useful, and how to create and use them in your Arduino projects.
What are Arduino Custom Libraries?
An Arduino custom library is a collection of code that provides additional functionality to the Arduino platform. These libraries are written in C++ and can be used to simplify complex tasks, add new features, or provide a higher level of abstraction for working with specific hardware components.
Custom libraries are created by the Arduino community and are available for download from various sources, including the official Arduino library manager and GitHub. They can be easily installed and used in the Arduino IDE, making it easy to incorporate them into your projects.
Why Use Arduino Custom Libraries?
There are several reasons why you might want to use Arduino custom libraries in your projects:
-
Simplify complex tasks: Custom libraries can provide high-level functions that simplify complex tasks, such as working with sensors, displays, or communication protocols. This can save you time and effort when developing your projects.
-
Add new features: Custom libraries can add new features to the Arduino platform that are not included in the core software. For example, you might use a custom library to add support for a specific sensor or communication protocol.
-
Improve code reusability: Custom libraries allow you to encapsulate common functionality into reusable modules that can be easily shared and used across multiple projects. This can help you write more modular and maintainable code.
-
Leverage community expertise: The Arduino community is a rich source of knowledge and expertise. By using custom libraries created by other members of the community, you can benefit from their experience and save time and effort in your own projects.
Creating an Arduino Custom Library
Creating an Arduino custom library is a straightforward process that involves the following steps:
-
Create a new directory: Create a new directory for your library in the Arduino libraries folder. The default location for this folder is
C:\Users\<username>\Documents\Arduino\libraries
on Windows or~/Documents/Arduino/libraries
on Mac and Linux. -
Create the library files: Inside the new directory, create the following files:
YourLibraryName.h
: This is the header file for your library, where you define the public interface of your library.YourLibraryName.cpp
: This is the implementation file for your library, where you define the actual functionality of your library.keywords.txt
: This file contains a list of keywords that should be highlighted in the Arduino IDE when using your library.-
examples
: This is a directory where you can provide example sketches that demonstrate how to use your library. -
Write the library code: In the
YourLibraryName.h
andYourLibraryName.cpp
files, write the code for your library. The header file should define the public interface of your library, while the implementation file should contain the actual functionality. -
Document your library: It’s important to provide clear and concise documentation for your library. This should include a README file that describes what your library does, how to install it, and how to use it. You should also provide comments in your code to explain how it works and what each function does.
-
Test your library: Before releasing your library to the public, it’s important to thoroughly test it to ensure that it works as expected. Create some example sketches that use your library and test them on different Arduino boards to ensure compatibility.
Example: Creating a Simple LED Library
Let’s walk through an example of creating a simple Arduino custom library that provides functions for controlling an LED.
First, create a new directory for your library called SimpleLED
in the Arduino libraries folder.
Inside the SimpleLED
directory, create the following files:
SimpleLED.h
:
#ifndef SimpleLED_h
#define SimpleLED_h
#include "Arduino.h"
class SimpleLED {
public:
SimpleLED(int pin);
void on();
void off();
void blink(int delay_ms);
private:
int _pin;
};
#endif
SimpleLED.cpp
:
#include "SimpleLED.h"
SimpleLED::SimpleLED(int pin) {
_pin = pin;
pinMode(_pin, OUTPUT);
}
void SimpleLED::on() {
digitalWrite(_pin, HIGH);
}
void SimpleLED::off() {
digitalWrite(_pin, LOW);
}
void SimpleLED::blink(int delay_ms) {
on();
delay(delay_ms);
off();
delay(delay_ms);
}
keywords.txt
:
SimpleLED KEYWORD1
on KEYWORD2
off KEYWORD2
blink KEYWORD2
examples/Blink/Blink.ino
:
#include <SimpleLED.h>
SimpleLED led(13);
void setup() {
// do nothing
}
void loop() {
led.blink(1000);
}
In this example, we define a SimpleLED
class that represents an LED connected to a specific pin. The class provides functions for turning the LED on and off, as well as a blink
function that blinks the LED with a specified delay.
The keywords.txt
file specifies which words in the library should be highlighted in the Arduino IDE.
The examples/Blink/Blink.ino
sketch demonstrates how to use the SimpleLED
library to blink an LED connected to pin 13.
Using Arduino Custom Libraries
Once you have created your Arduino custom library, using it in your sketches is easy. Here’s how to do it:
-
Install the library: If you haven’t already done so, copy your library directory to the Arduino libraries folder.
-
Include the library in your sketch: To use your library in a sketch, you need to include it at the top of your sketch using the
#include
directive. For example:
#include <YourLibraryName.h>
- Use the library functions: Once you have included your library, you can use its functions in your sketch just like any other Arduino function. Refer to your library’s documentation for details on how to use each function.
Example: Using the SimpleLED Library
Here’s an example sketch that demonstrates how to use the SimpleLED
library we created earlier:
#include <SimpleLED.h>
SimpleLED led1(13);
SimpleLED led2(12);
void setup() {
// do nothing
}
void loop() {
led1.on();
led2.off();
delay(1000);
led1.off();
led2.on();
delay(1000);
}
In this sketch, we create two SimpleLED
objects, led1
and led2
, which represent LEDs connected to pins 13 and 12, respectively. In the loop
function, we alternately turn on and off each LED with a delay of one second.
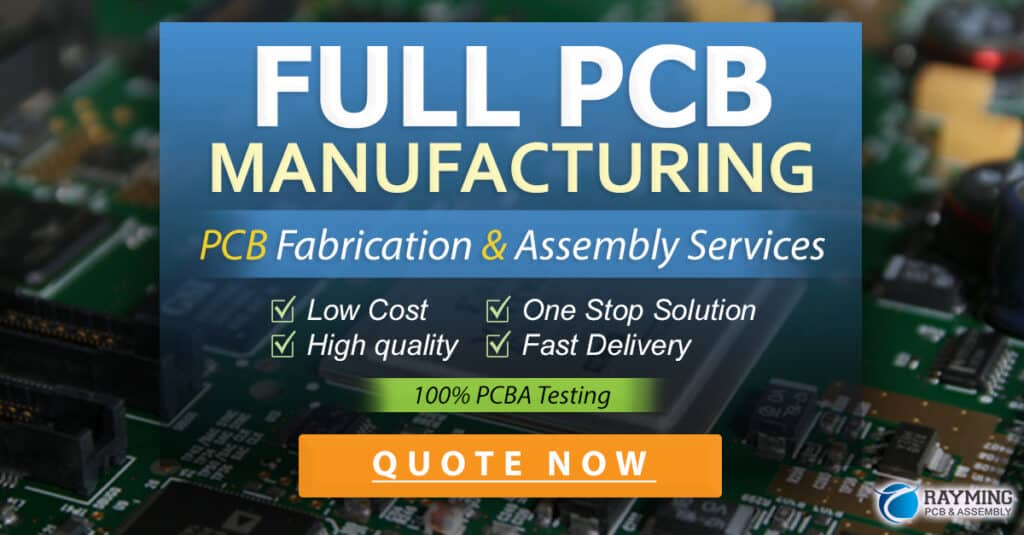
Popular Arduino Custom Libraries
There are many popular Arduino custom libraries available that provide a wide range of functionality. Here are a few examples:
Library | Description |
---|---|
Wire | Allows communication with I2C / TWI devices |
SPI | Allows communication with SPI devices |
Servo | Allows control of servo motors |
LiquidCrystal | Allows control of LCD displays |
DHT | Allows reading of temperature and humidity from DHT sensors |
NeoPixel | Allows control of NeoPixel LED strips |
WiFi | Allows communication with WiFi networks |
Ethernet | Allows communication with Ethernet networks |
These are just a few examples of the many libraries available. The Arduino community is constantly creating new libraries to add functionality and support new hardware.
Frequently Asked Questions (FAQ)
-
What is an Arduino custom library?
An Arduino custom library is a collection of code that provides additional functionality to the Arduino platform. These libraries are written in C++ and can be used to simplify complex tasks, add new features, or provide a higher level of abstraction for working with specific hardware components. -
How do I install an Arduino custom library?
To install an Arduino custom library, simply copy the library directory to the Arduino libraries folder. The default location for this folder isC:\Users\<username>\Documents\Arduino\libraries
on Windows or~/Documents/Arduino/libraries
on Mac and Linux. -
How do I use an Arduino custom library in my sketch?
To use an Arduino custom library in your sketch, you need to include it at the top of your sketch using the#include
directive. For example:#include <YourLibraryName.h>
. Once you have included the library, you can use its functions just like any other Arduino function. -
Where can I find Arduino custom libraries?
Arduino custom libraries can be found in various places, including the official Arduino library manager, GitHub, and other online resources. The Arduino community is constantly creating and sharing new libraries, so there are always new libraries to explore. -
How can I contribute to the Arduino custom library ecosystem?
If you have created an Arduino custom library that you think would be useful to others, you can share it with the community by publishing it on GitHub or other online platforms. You can also contribute to existing libraries by submitting bug reports, feature requests, or pull requests on the library’s repository.
Conclusion
Arduino custom libraries are a powerful tool for extending the functionality of the Arduino platform. By using custom libraries, you can simplify complex tasks, add new features, and leverage the expertise of the Arduino community.
Creating your own custom libraries is a straightforward process that involves creating the necessary files, writing the library code, documenting your library, and testing it thoroughly. Once your library is ready, you can easily use it in your sketches by including it at the top of your sketch and using its functions.
There are many popular Arduino custom libraries available that provide a wide range of functionality, from communication protocols to sensor libraries to LED control libraries. By exploring these libraries and contributing to the Arduino custom library ecosystem, you can take your Arduino projects to the next level and help others do the same.
Leave a Reply